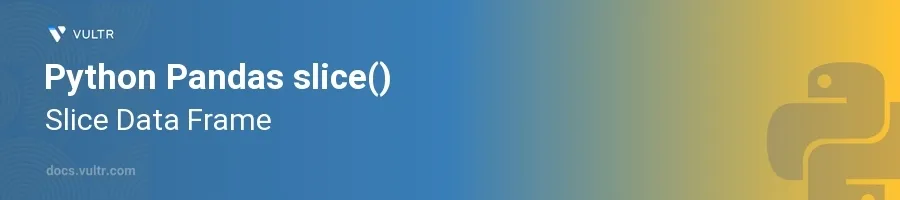
Introduction
The slice()
function in Python's Pandas library is a versatile tool for selecting specific sections of data from a DataFrame. This function is particularly useful when you need to work with subsets of large datasets for analysis, visualization, or further processing. By mastering the slice()
method, you enhance your data handling and analytical capabilities in Python.
In this article, you will learn how to utilize the slice()
function to effectively slice DataFrames. Explore the application of this function in various contexts to retrieve rows and columns, to aid in breaking down your data analysis tasks into manageable pieces.
Slicing Rows in a DataFrame
Select a Range of Rows
Import the Pandas library and create a DataFrame.
Use the
slice()
function to specify the range of rows.pythonimport pandas as pd data = { 'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Edward'], 'Age': [25, 30, 35, 40, 45] } df = pd.DataFrame(data) row_slice = slice(1, 4) sliced_df = df[row_slice] print(sliced_df)
This script creates a DataFrame with names and ages and then slices it from the second row (index 1) to the fourth row (index 3), inclusive of the start index and exclusive of the stop index. The result is a subset of the original DataFrame containing rows for Bob, Charlie, and David.
Using slice() with a Step Value
Apply the
slice()
function with a step parameter to select every nth row.pythonstep_slice = slice(0, 5, 2) stepped_df = df[step_slice] print(stepped_df)
The
step_slice
specifies that rows should be selected from the start to the 5th index, skipping every second row. This operation returns rows for Alice, Charlie, and Edward.
Slicing Columns in a DataFrame
Select Specific Columns
Understand that direct
slice()
usage on columns requires.loc
or.iloc
.Define a slice for the columns desired.
pythoncolumn_slice = df.loc[:, slice('Name', 'Age')] print(column_slice)
This example uses
loc
to slice all rows (indicated by:
) and selects all columns between 'Name' and 'Age' inclusively. Since in this instance all columns are included in the slice, the entire DataFrame is displayed.
Using Conditions to Slice Columns
Combine
slice()
with conditions to filter both rows and columns.pythonconditional_slice = df.loc[df['Age'] > 30, slice('Name')] print(conditional_slice)
Here, the DataFrame is sliced to show only the 'Name' column for entries where the age is greater than 30, which applies to Charlie, David, and Edward.
Conclusion
By incorporating the slice()
function into your Python Pandas workflows, you'll find it easier to manage and analyze slices of data from larger DataFrames. Whether selecting specific rows, every nth entry, or bounding column selections, the slice()
method offers a straightforward way to access and manipulate subsets of data efficiently. Foster a deeper understanding of this functionality to enhance your data handling tasks and streamline your analytical projects.
No comments yet.