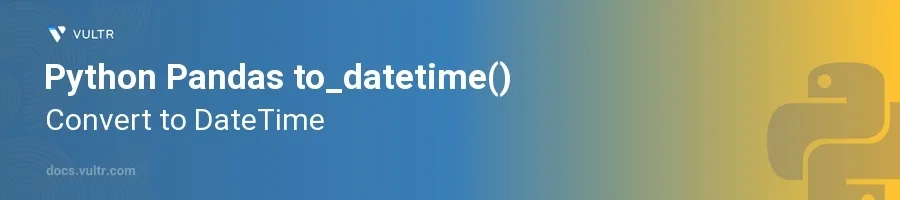
Introduction
The to_datetime()
function in Python's Pandas library is a versatile tool for converting various date and time formats into pandas DateTime objects. This capability is essential for data analysis, especially when dealing with time-series data where date and time manipulations are frequent operations. The function can handle a wide array of string formats and can also convert entire arrays or DataFrame columns to datetime.
In this article, you will learn how to leverage the to_datetime()
function effectively in various scenarios. Explore techniques for converting single strings, lists of strings, and Series objects to pandas DateTime objects. Understand different parameter settings that customize how dates and times are parsed, especially in cases of ambiguous formats or missing data.
Converting Single Date Strings
Convert a Basic Date String
Start with a simple date string.
Use
to_datetime()
to convert it to a DateTime object.pythonimport pandas as pd date_str = '2023-01-01' date_time_obj = pd.to_datetime(date_str) print(date_time_obj)
This snippet transforms the string
'2023-01-01'
into a DateTime object. The printed result shows the date with a default time set to00:00:00
.
Handle Different Date Formats
Handle a date string in a non-standard format.
Specify the format to ensure correct parsing.
pythondate_str = '01-31-2023' date_time_obj = pd.to_datetime(date_str, format='%m-%d-%Y') print(date_time_obj)
By providing the format parameter (
format='%m-%d-%Y'
), ensure that the parser interprets the date correctly, avoiding misinterpretation or errors.
Converting Lists and Series
Convert a List of Date Strings
Create a list containing date strings.
Utilize
to_datetime()
to convert the entire list.pythonlist_dates = ['2023-01-01', '2023-01-02', '2023-01-03'] datetime_objs = pd.to_datetime(list_dates) print(datetime_objs)
This code converts each string in the list to a DateTime object, resulting in a DatetimeIndex object containing all the converted dates.
Convert a Pandas Series
Convert a column in a DataFrame or a Series containing date strings to DateTime objects.
Apply
to_datetime()
directly to the Series.pythonseries_dates = pd.Series(['2023-01-01', '2023-02-01', '2023-03-01']) datetime_objs = pd.to_datetime(series_dates) print(datetime_objs)
Similar to converting a list, this snippet processes a pandas Series, turning each element into a DateTime object.
Dealing with Ambiguous and Missing Data
Parse Ambiguous Dates
Address ambiguous date formats where day and month could be confused.
Use the
dayfirst
parameter to clarify the order.pythonambiguous_date = '01-02-2023' # Could be Jan 2 or Feb 1 date_time_obj = pd.to_datetime(ambiguous_date, dayfirst=True) print(date_time_obj)
Setting
dayfirst=True
instructs pandas to interpret the first part of the date as the day, making the parsing unambiguous.
Handle Missing or Faulty Date Entries
Manage datasets with missing or faulty date entries effectively.
Utilize the
errors
parameter to control the output upon encountering bad data.pythonfaulty_dates = ['2023-01-01', 'not a date', '2023-01-02'] datetime_objs = pd.to_datetime(faulty_dates, errors='coerce') print(datetime_objs)
Using
errors='coerce'
turns any problematic entries intoNaT
(Not a Time), ensuring the continuity of data processing without interruptions from parsing errors.
Conclusion
The to_datetime()
function in pandas is a powerful and flexible tool for converting strings, lists, or Series to DateTime objects, facilitating the manipulation and analysis of time-series data. Understand and apply this function in various contexts, from simple string conversions to handling ambiguous formats and missing data points. By utilizing the techniques discussed, you streamline data pre-processing tasks and enhance the robustness and accuracy of your data analysis workflows.
No comments yet.