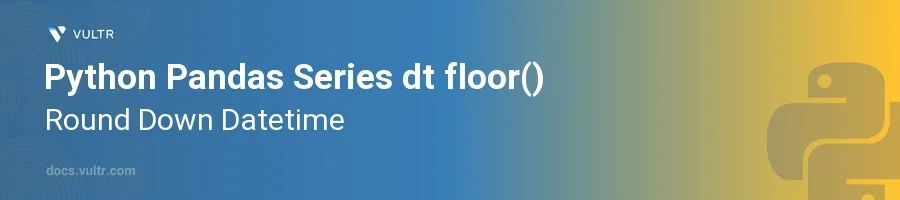
Introduction
The floor()
method in Python's Pandas library is a powerful tool for manipulating datetime data in a Series object. This function rounds down datetime values to a specified date frequency, making it particularly useful when normalizing or summarizing time series data. By rounding down, you ensure that all dates in your data fit into regular intervals, a common requirement for time-series analyses where consistency and order are crucial.
In this article, you will learn how to use the dt.floor()
method on a Series object containing datetime values. Explore how to round down these datetimes to various frequencies like days, hours, or even minutes, and see practical examples that demonstrate how to implement these techniques effectively.
Understanding the dt.floor() Method
The dt
accessor in Pandas allows us to work specifically with timedata in Series and DataFrame objects. The floor()
method is part of this datetime accessor, enabling detailed time frequency adjustments.
Basics of the dt.floor() Method
Start by importing the Pandas library and creating a datetime Series.
Apply the
dt.floor()
method to round down these datetime values.pythonimport pandas as pd # Create a datetime Series s = pd.Series(pd.date_range('2023-01-01 14:45:30', periods=4, freq='T')) # Apply dt.floor() to round down the datetimes floor_series = s.dt.floor('H') print(floor_series)
This code generates a Series of datetime values every minute, starting from '2023-01-01 14:45:30' and uses
dt.floor('H')
to round down these values to the nearest hour. The output will show all datetime entries normalized to '2023-01-01 14:00:00'.
Rounding to Specific Frequencies
Understand that
dt.floor()
can handle various frequencies: 'H' for hours, 'D' for days, 'T' (or 'min') for minutes, and more.Create a series with datetime data.
Use different granularity levels in the
dt.floor()
method to see the effect.python# Applying different frequencies to dt.floor() day_floor = s.dt.floor('D') minute_floor = s.dt.floor('T') print("Day Floor:\n", day_floor) print("Minute Floor:\n", minute_floor)
This example highlights how you can round down to the nearest day and minute. The day rounding will adjust all timestamps to the start of their respective days, while minute rounding simply removes seconds from the time.
Advanced Uses of dt.floor()
In real-world applications, rounding time series data helps in generating reports, performing group-by operations, or preparing data for further statistical analysis.
Floor in Time Series Grouping Operations
Consider a time series data set where entries are recorded every minute.
Use
dt.floor()
for daily grouping and summing of data.pythonimport numpy as np # Generating time series data rng = pd.date_range('2021-01-01', periods=1440, freq='T') data = pd.Series(np.random.randn(1440), index=rng) # Group by day and summarize grouped = data.groupby(data.index.floor('D')).sum() print(grouped)
This example aggregates data by the day, using
floor('D')
to create daily bins even if time stamps are detailed to the minute within the original data. Such grouping is essential in various analytical tools used in finance, meteorology, or marketing analytics.
Conclusion
Mastering the dt.floor()
function in Pandas is fruitful for anyone dealing with datetime data in Python. It provides a systematic approach to down-sampling or normalizing datetime objects to specific frequencies. Use these methods to ensure your data aligns with regular intervals, which is invaluable in analysis involving temporal patterns. By applying the insights and techniques from this discussion, you advance the clarity and precision of your time-series handling in Python scripts, making your data preparation tasks far more manageable and accurate.
No comments yet.