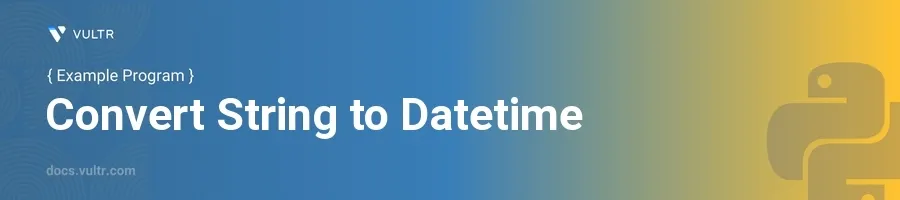
Introduction
Python's ability to handle dates and time is crucial for data analysis, web development, and automating tasks, among other applications. One common requirement in programming is converting strings into datetime objects to perform operations like comparisons, arithmetic, formatting, or simply extracting specific components like the year, month, or day.
In this article, you will learn how to convert a string into a datetime object in Python using the datetime
module. Explore practical examples that demonstrate converting various formatted strings into datetime objects, adjusting for time zones, and handling common errors during conversion.
Basic Conversion of String to Datetime
Convert Using strptime()
Import the
datetime
module.Define a string that represents a date.
Use the
datetime.strptime()
method to convert the string into a datetime object.pythonfrom datetime import datetime date_string = "2023-01-25" date_object = datetime.strptime(date_string, "%Y-%m-%d") print(date_object)
This code snippet converts the string
"2023-01-25"
into a datetime object. The format string"%Y-%m-%d"
tells Python how to interpret the date.
Handling Different Date Formats
Prepare to convert various date formats by understanding Python's datetime directives.
Convert different formatted strings, such as data including time or different date separators.
pythondatetime_str1 = "25/01/2023" datetime_str2 = "01-25-2023 14:30" date_object1 = datetime.strptime(datetime_str1, "%d/%m/%Y") date_object2 = datetime.strptime(datetime_str2, "%m-%d-%Y %H:%M") print(date_object1) print(date_object2)
In these examples, different string formats are parsed by specifying the correct format, such as day before month or including time.
Advanced Usage
Time Zone Handling
Use Python's
pytz
module to handle time zone conversions.Convert a string with a time zone specification to a timezone-aware datetime object.
pythonimport pytz timezone_str = "2023-01-25 15:00:00+0200" timezone_format = "%Y-%m-%d %H:%M:%S%z" tz_aware_datetime = datetime.strptime(timezone_str, timezone_format) tz_aware_datetime = tz_aware_datetime.astimezone(pytz.utc) print(tz_aware_datetime)
This snippet demonstrates converting a string with a timezone offset into a UTC datetime object with the use of
pytz
.
Error Handling in Date Conversion
Anticipate and manage errors that may occur when the date format is incorrect.
Use a
try-except
block to gracefully handle parsing errors.pythontry: wrong_date_str = "2023-30-01" attempt_conversion = datetime.strptime(wrong_date_str, "%Y-%d-%m") print(attempt_conversion) except ValueError as e: print(f"Error occurred: {e}")
This script attempts to parse a string with an incorrect date format and handles any
ValueError
by printing an error message.
Conclusion
Converting strings into datetime objects in Python is an essential task for many applications involving date manipulations. By using Python's datetime.strptime()
method, you can handle various date string formats efficiently. Remember to consider timezone handling and error management to cover common pitfalls in date conversion processes. Implement these conversion techniques in your Python projects to manage date and time data more robustly and effectively.
No comments yet.