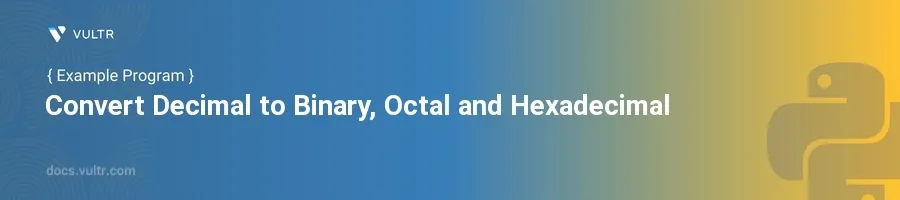
Introduction
Converting decimal numbers into binary, octal, and hexadecimal forms is a common task in programming, especially when dealing with low-level operations. These forms are pivotal in understanding computer architecture, data storage, and processing, as they represent different number systems used by computers.
In this article, you will learn how to convert decimal numbers to binary, octal, and hexadecimal formats using Python. Through practical examples, explore Python's built-in functions to handle these conversions efficiently.
Converting Decimal to Binary
Convert Using bin()
Obtain the decimal number that needs to be converted.
Utilize the
bin()
function to convert the decimal to binary.pythondecimal_number = 34 binary_output = bin(decimal_number) print(binary_output)
This code snippet converts the decimal number
34
into a binary string. Python’sbin()
function returns the binary representation prefixed with '0b'.
Understanding the Output
bin()
outputs a string that starts with '0b', denoting that the following digits are in binary format.- For a cleaner output without the prefix, strip the first two characters with slicing:
binary_output[2:]
.
Converting Decimal to Octal
Convert Using oct()
Capture the decimal number for conversion.
Apply the
oct()
function to convert it to octal.pythondecimal_number = 34 octal_output = oct(decimal_number) print(octal_output)
In this example, the decimal number
34
is converted to an octal format. Theoct()
function also provides the output prefixed with '0o'.
Understanding the Output
- The representation starts with '0o', indicating octal.
- Use slicing for format without the prefix by using
octal_output[2:]
.
Converting Decimal to Hexadecimal
Convert Using hex()
Start with a specific decimal number.
Utilize the
hex()
function for hexadecimal conversion.pythondecimal_number = 34 hexadecimal_output = hex(decimal_number) print(hexadecimal_output)
This snippet converts the decimal number
34
into hexadecimal. The result includes a prefix '0x' which denotes hexadecimal format.
Understanding the Output
- The hexadecimal representation includes '0x'.
- For output without '0x', employ slicing:
hexadecimal_output[2:]
.
Conclusion
Converting numbers between decimal, binary, octal, and hexadecimal formats in Python is straightforward thanks to the built-in functions bin()
, oct()
, and hex()
. These functions return strings that are easy to manipulate if you need to remove system-specific prefixes. Utilize these techniques to effectively manage number system conversions in your applications, enhancing both flexibility and efficiency.
No comments yet.