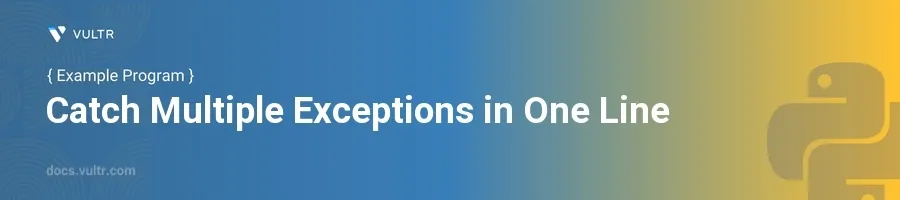
Introduction
Handling exceptions in Python is a fundamental aspect of writing clean, robust, and user-friendly code. Multiple exceptions may occur, and how you handle these can significantly impact the user experience and code maintainability. Catching multiple exceptions efficiently in one line can streamline error handling in programs where different exceptions may be raised for similar reasons.
In this article, you will learn how to catch multiple exceptions in a single line using Python. This guide also covers how to handle multiple exceptions in Python using various try-except techniques, including one-line solutions.
This approach simplifies error handling management by consolidating multiple error checks into a concise format. You'll see practical examples that demonstrate how to use this technique in various scenarios.
Catching Multiple Exceptions in One Block
Basic Syntax to Catch Multiple Exceptions in Python
Use the try-except statement to check for exceptions.
Catch multiple exceptions by grouping them within a tuple.
pythontry: # code that might throw multiple distinct exceptions x = 1 / 0 except (ZeroDivisionError, KeyError, TypeError) as e: print(f"Caught an exception: {e}")
This snippet shows how to catch multiple exceptions in Python efficiently. It handles three different types of exceptions in a single line. If any of the
ZeroDivisionError
,KeyError
, orTypeError
occur, the code inside the except block will execute.
Handling Exceptions with Similar Responses
Recognize scenarios where multiple exceptions should result in similar outcomes.
Group these exceptions together to tidy up the code.
pythontry: # code that might throw exceptions data = {'key': 'value'} print(data['non_existent_key']) except (KeyError, IndexError) as e: print(f"Handling missing key or index error: {e}")
Here, both
KeyError
(missing dictionary key) andIndexError
(out-of-range list index) are handled by the same piece of code, reflecting their similar impact on the program.
Complex Conditional Handling in Python
Sometimes, similar error types need slightly different handling.
Still group errors, but use additional conditions to tweak the response.
pythontry: result = 10 / 0 except (ZeroDivisionError, OverflowError) as e: if isinstance(e, ZeroDivisionError): print("Cannot divide by zero") else: print("Math calculation overflow")
In this code,
ZeroDivisionError
andOverflowError
are caught together, but the response differs slightly depending on the type of exception. This is a useful way to handle multiple exceptions in Python when different errors require specific responses.
Using One-Line Try Except in Python
To catch multiple exceptions in Python in a compact form, you can use the one-line
try-except
syntax.This is especially useful for handling minor errors without breaking the program flow.
pythontry: x = 1 / 0 except (ZeroDivisionError, ValueError): print("Error occurred")
This one-line
try-except
statement catches multiple exceptions and provides a concise error-handling method. This is an efficient approach when you need to manage multiple exceptions in Python without using a multi-line block.
Best Practices for Exception Handling
- Be Specific: Catch only those exceptions that you expect and know how to handle.
- Log Detailed Errors: Especially in production code, log errors comprehensively.
- Avoid General Exceptions: Catching
Exception
orBaseException
can sometimes mask other bugs.
Conclusion
Catching multiple exceptions in one line in Python simplifies and organizes exception handling. It allows combining the handling of different error types when they warrant similar user feedback or corrective measures. With the techniques discussed, enhance your error management strategy to make your applications more error-resistant and easier to maintain. By tailoring your exception handling strategy as shown, you ensure better error recovery and contribute to a smoother user experience.
No comments yet.