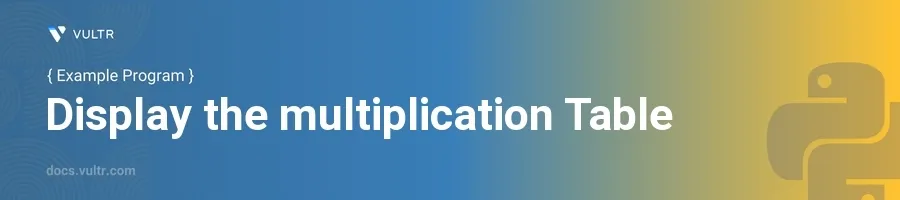
Introduction
Python offers an intuitive way to perform and display mathematical operations, such as generating a multiplication table. Multiplication tables are fundamental in learning arithmetic and are commonly used in educational settings to help students understand and visualize the process of multiplication. These tables also serve as a practical example of nested loops in programming.
In this article, you will learn how to create a Python program to display the multiplication table. Through clear examples, understand how loops work in generating these tables, and see how to format the output to make the tables easy to read.
Displaying a Basic Multiplication Table in Python
Define the Range and Multiplicand
Start by deciding the size of the multiplication table - typically, tables go from 1 to 10.
Set the number (multiplicand) for which you want the multiplication table.
pythonmultiplicand = 5 for i in range(1, 11): result = multiplicand * i print(f"{multiplicand} x {i} = {result}")
This script prints the multiplication table for 5. The loop iterates through numbers 1 to 10, multiplying each by 5 and printing the result.
Format the Output for Clarity
Ensure the output is well-formatted so that the numbers align properly, making the table easy to read, especially for larger tables.
pythonmultiplicand = 5 for i in range(1, 11): result = multiplicand * i print(f"{multiplicand:2} x {i:2} = {result:2}")
Here,
:2
in the format string ensures that numbers align correctly by allotting 2 spaces for each number.
Creating a Full Multiplication Table Program in Python Using For Loop
Generate a Table for All Numbers up to a Limit
To display a complete multiplication table, use nested loops—one to iterate through the base numbers and another for the multipliers.
pythonfor multiplicand in range(1, 11): for multiplier in range(1, 11): result = multiplicand * multiplier print(f"{multiplicand} x {multiplier} = {result}", end='\t') print() # Adds a newline after each row
This example uses a nested loop to generate a complete 10x10 multiplication table. The
end='\t'
adds a tab after each multiplication result for better spacing, and theprint()
at the end of the outer loop inserts a newline after each row.
Enhance Readability with Headers
Add headers to the table to clearly define multipliers and multiplicands.
pythonprint(" ", end='') for header in range(1, 11): print(f"{header:2}", end=' ') print() print(" " + ("--- " * 10)) for multiplicand in range(1, 11): print(f"{multiplicand:2} | ", end='') for multiplier in range(1, 11): result = multiplicand * multiplier print(f"{result:2}", end=' ') print()
This script enhances the table by adding a top row of headers (1-10) and a column on the left for each row's multiplicand. The headers help distinguish the values for easy reference.
Python Program to print multiplication table of a given number
Here's a simple Python program to print the multiplication table of a given number:
# Take user input
num = int(input("Enter a number: "))
print(f"\nMultiplication Table of {num}\n")
# Loop from 1 to 10 to generate the table
for i in range(1, 11):
print(f"{num} x {i} = {num * i}")
Explanation
Takes input from the user.
Loops through 1 to 10 to multiply the number.
Prints the results in a formatted way using f-strings.
Conclusion
Creating a multiplication table in Python is an excellent exercise for understanding loops and formatting output. By implementing the given examples, you can generate tables of any size and style them for better readability. Use these techniques to create versatile and user-friendly multiplication applications for educational or practice purposes in Python programming. Use various configurations and formatting options to customize the table according to your needs.
No comments yet.