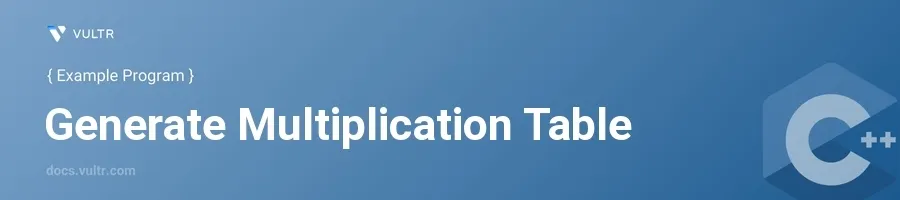
Introduction
A multiplication table is a valuable tool for learning and practicing basic arithmetic operations. It lists the products of pairs of numbers, typically one to ten or more, making it easier to understand and memorize multiplication facts. In programming, creating a multiplication table can also serve as an engaging way to work with loops, functions, and output formatting.
In this article, you will learn how to generate a multiplication table through a C++ program. You will see examples using loops to compute and exhibit the tables dynamically, catering to any size specified by the user.
Generating a Basic Multiplication Table
Establishing the Loop Structure
Start by including the necessary header files.
Prompt the user to enter the size of the multiplication table.
Use nested loops to iterate through the numbers and calculate the products.
cpp#include<iostream> int main() { int n; std::cout << "Enter the size of the multiplication table: "; std::cin >> n; for (int i = 1; i <= n; i++) { for (int j = 1; j <= n; j++) { std::cout << i * j << "\t"; } std::cout << std::endl; } return 0; }
In this example, the program first includes the
<iostream>
header to handle input and output operations. It then prompts the user to enter the size of the multiplication table. The nestedfor
loops calculate the product ofi
andj
for each pair, and the results are displayed in a tabular format using the tab character\t
for better readability.
Improving Output Readability
Add rows and column headings to make the table more understandable.
Modify the loops to print the headers and adjust spacing according to the numbers.
cpp#include<iostream> #include<iomanip> // For std::setw() int main() { int n; std::cout << "Enter the size of the multiplication table: "; std::cin >> n; // Printing the top header row std::cout << std::setw(5) << "*"; for (int j = 1; j <= n; j++) { std::cout << std::setw(5) << j; } std::cout << std::endl; for (int i = 1; i <= n; i++) { std::cout << std::setw(5) << i; // Print the row header for (int j = 1; j <= n; j++) { std::cout << std::setw(5) << i * j; } std::cout << std::endl; } return 0; }
This enhancement introduces the
<iomanip>
header to utilize thestd::setw()
function, which sets the width of each output field, ensuring that the values align correctly regardless of their number of digits. The table now starts with a header row and a header column that indicate which numbers are being multiplied, making it more user-friendly.
Advanced Customizations
Adding Functionality to Print Tables for Specific Ranges
Modify the program to accept the starting and ending numbers for the table.
Adjust the loop boundaries according to the user input.
cpp#include<iostream> #include<iomanip> int main() { int start, end; std::cout << "Enter the starting number of the table: "; std::cin >> start; std::cout << "Enter the ending number of the table: "; std::cin >> end; // Print headers std::cout << std::setw(5) << "*"; for (int j = start; j <= end; j++) { std::cout << std::setw(5) << j; } std::cout << std::endl; for (int i = start; i <= end; i++) { std::cout << std::setw(5) << i; for (int j = start; j <= end; j++) { std::cout << std::setw(5) << i * j; } std::cout << std::endl; } return 0; }
This version of the program allows for more flexibility. Users can specify any range of numbers (positive or negative) for which they need the multiplication table. This feature is particularly useful for educational purposes or for customized calculations.
Conclusion
Building a multiplication table in C++ offers a clear perspective on how nested looping works, as well as how to manage simple input/output operations and output formatting. By meticulously organizing and customizing the output layout, ensure that the data presentation is clear and useful. Whether for educational applications or as a functionality in larger software solutions, the skills covered here give solid foundational knowledge in creating practical and dynamic programming solutions.
No comments yet.