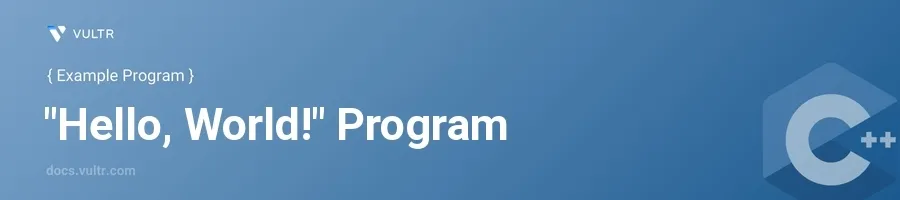
Introduction
The "Hello, World!" program is often the first step for beginners learning how to code in C++. This simple program outputs the text "Hello, World!" to the console, serving as a basic snippet that showcases the structure and syntax of a C++ program.
In this article, you will learn how to write and understand the "Hello, World!" program in C++. The examples provided will guide you through writing your first C++ program and understanding each part of the code involved.
Writing Your "Hello, World!" Program in C++
Setting Up the Basic Structure
Start with including the necessary headers.
Define the main function.
cpp#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
In this code snippet:
#include <iostream>
includes the Standard Input Output streams header, which contains definitions for input/output operations.int main()
defines the main function, where the execution of the program begins.std::cout << "Hello, World!" << std::endl;
prints "Hello, World!" followed by a newline.return 0;
signifies that the program ended successfully.
Understanding the Output Statement
Focus on the line that handles the output.
Here,
std::cout
is the standard character output stream in C++. It is used with the insertion operator (<<
) to write to the standard output, which is usually the console.std::endl
is an manipulator which inserts a newline character and flushes the stream.cppstd::cout << "Hello, World!" << std::endl;
This statement outputs the string "Hello, World!" followed by a new line.
Conclusion
The "Hello, World!" program in C++ is a beginner's entry point into understanding the syntax and structure of a C++ program. By creating this simple program, you familiarize yourself with the use of headers, primary functions, output operations, and proper program termination. Implement this program as your first step into the C++ programming world, refining your understanding of basic coding concepts.
No comments yet.