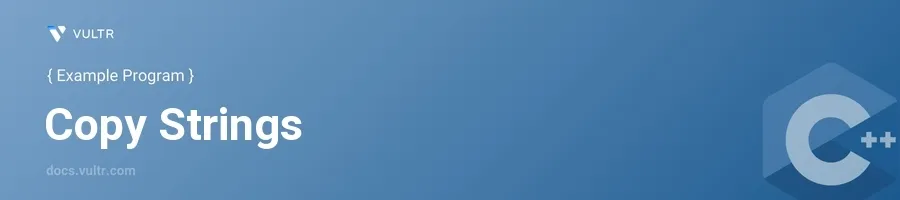
Introduction
Copying strings is a fundamental operation in C++ programming, involving the duplication of data from one string variable to another. This operation can be performed using various methods in C++, including the use of standard string functions, character arrays, or modern C++ techniques.
In this article, you will learn how to copy strings in C++ through multiple examples and methods. Discover the differences between using traditional character arrays and the more modern string class provided by the C++ Standard Library, and see how these techniques apply in practical programming scenarios.
Copying Strings Using the std::string Class
Direct Assignment
Initialize a string variable with a given value.
Copy the string to another string variable using the assignment operator.
cpp#include <iostream> #include <string> int main() { std::string original = "Hello, world!"; std::string copy = original; std::cout << "Original: " << original << std::endl; std::cout << "Copy: " << copy << std::endl; return 0; }
This example demonstrates how
original
string is directly assigned tocopy
. The output shows that both strings contain the same value, proving that the copy was successful.
Using the copy() Method
Use the
copy()
method from the std::string class to copy characters into a character array.Specify the number of characters to copy and the starting position.
cpp#include <iostream> #include <string> int main() { std::string original = "Hello, world!"; char buffer[20]; original.copy(buffer, original.length(), 0); buffer[original.length()] = '\0'; // Null-terminate the character array std::cout << "Buffer contains: " << buffer << std::endl; return 0; }
In this snippet,
copy()
is used to transfer characters fromoriginal
tobuffer
. The buffer is then null-terminated to ensure that it represents a proper C-style string.
Copying Strings Using Character Arrays
Using strcpy()
Include the
<cstring>
header for string manipulation functions.Use the
strcpy()
function to copy the contents from one character array to another.cpp#include <iostream> #include <cstring> // For strcpy int main() { char original[] = "Hello, world!"; char copy[50]; strcpy(copy, original); std::cout << "Original: " << original << std::endl; std::cout << "Copy: " << copy << std::endl; return 0; }
The
strcpy()
function copies the string fromoriginal
tocopy
including the terminating null character automatically.
Using a Manual Loop
Copy each character from the source to the destination manually using a loop.
Ensure to null-terminate the destination array after copying all characters.
cpp#include <iostream> int main() { char original[] = "Hello, world!"; char copy[50]; int i; for(i = 0; original[i] != '\0'; i++) { copy[i] = original[i]; } copy[i] = '\0'; // Null-terminate the array std::cout << "Original: " << original << std::endl; std::cout << "Copy: " << copy << std::endl; return 0; }
This code manually iterates through
original
and copies each character tocopy
. The loop stops when it encounters the null character, which it then adds to the end ofcopy
.
Conclusion
With multiple ways to copy strings in C++, choose the method that best fits the scenario and the data structures being used. The std::string
class provides a flexible and safe way to handle strings in modern C++, while traditional character arrays offer a more manual approach, often used where fine control over memory and performance is crucial. Apply these techniques to ensure that your C++ programs handle string duplication efficiently and correctly.
No comments yet.