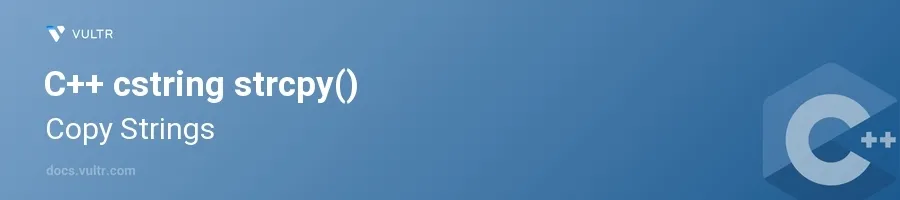
Introduction
The strcpy()
function in C++ is a standard library function used for copying strings from one character array to another. This function resides within the <cstring>
header and is foundational in many programs that require manipulation of C-style strings. Operating correctly with strcpy()
is crucial, especially considering the vulnerabilities associated with improper string handling, such as buffer overflow.
In this article, you will learn how to safely and effectively use the strcpy()
function. The discussion will include examples of basic string copying, handling strings in different scenarios, and the potential pitfalls of using this function incorrectly.
Basic Usage of strcpy()
Copying a Simple String
Include the
<cstring>
header in your C++ program.Declare two character arrays: one as the source and one as the destination.
Use
strcpy()
to copy the source string to the destination string.cpp#include <cstring> char src[] = "Hello, World!"; char dest[50]; // Make sure the destination is large enough strcpy(dest, src);
This snippet copies the contents of
src
intodest
. Ensure the destination array is large enough to hold the source string along with the null-terminator character.
Proper Buffer Size Management
Always ensure the destination buffer is large enough to store the source string including its null terminator.
If the size is underestimated, it risks buffer overflow, leading to crashes or vulnerabilities.
cppchar shortBuffer[5]; strcpy(shortBuffer, "Too long string");
In this faulty example, buffer overflow occurs because
shortBuffer
can only accommodate 5 characters, including the null terminator, but the source string exceeds this limit.
Handling Strings in Structs
Copying Strings to Struct Members
Define a struct with a character array for storing strings.
Use
strcpy()
to copy a string into the struct's string member.cpp#include <cstring> struct Person { char name[100]; }; Person person; strcpy(person.name, "John Doe");
This code initializes a
Person
struct and then usesstrcpy()
to copy the name "John Doe" into thename
field of the struct.
Preventing Common Pitfalls
Avoiding Buffer Overflow
Carefully analyze the size of the destination buffer before copying.
Consider using
strncpy()
as a safer alternative, which limits the number of characters copied.cppchar src[] = "Example"; char dest[10]; strncpy(dest, src, sizeof(dest) - 1); dest[sizeof(dest) - 1] = '\0'; // Ensure null termination
This code uses
strncpy()
to prevent buffer overflow by explicitly specifying the maximum number of characters to copy. It also ensures that the string is null-terminated.
Conclusion
The strcpy()
function in C++ is essential for copying strings but requires cautious handling to ensure safety and efficiency. Always verify that your destination buffer is large enough to accommodate the entire string you wish to copy, including the null terminator. For better safety against buffer overflow, consider using strncpy()
and explicitly manage the string termination. Use the examples and strategies discussed here to harness the capability of strcpy()
while mitigating potential risks in your C++ applications.
No comments yet.