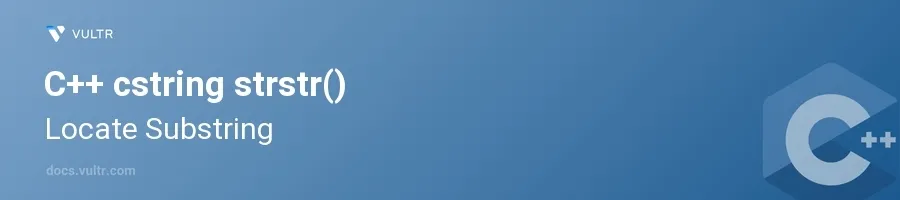
Introduction
The strstr()
function in C++ is a powerful utility in the C-style string manipulation arsenal, allowing developers to locate a substring within another string. This function is part of the <cstring>
header and provides the functionality to search for a sequence of characters, effectively helping in tasks such as parsing and data validation.
In this article, you will learn how to skillfully use the strstr()
function to locate a substring within a string. Explore practical examples demonstrating how to apply this function within different contexts, and understand how to handle its output to make informed decisions in your code.
Basic Usage of strstr()
Locating a Simple Substring
Initialize a C-style string (character array) that you'll search.
Define the substring you want to find.
Use
strstr()
to search for the substring in the main string.cpp#include <cstring> #include <iostream> int main() { const char* mainString = "Hello, world!"; const char* subString = "world"; char* result = strstr(mainString, subString); if (result) { std::cout << "Substring found: " << result << std::endl; } else { std::cout << "Substring not found." << std::endl; } return 0; }
This example searches for the substring "world" in "Hello, world!". If found, it prints the substring and all characters that follow.
Handling Cases Where the Substring Is Not Found
Properly check if
strstr()
returnsnullptr
which indicates that the substring was not found in the main string.cppconst char* mainString = "Hello, C++ programmers!"; const char* subString = "Java"; char* result = strstr(mainString, subString); if (result) { std::cout << "Substring found: " << result << std::endl; } else { std::cout << "Substring not found." << std::endl; }
This snippet demonstrates
strstr()
usage when the substring does not exist in the main string. By checking fornullptr
, you control the flow of the program to handle such cases gracefully.
Advanced Uses of strstr()
Finding Multiple Occurrences
Iterate through the main string, using
strstr()
to find subsequent occurrences of the substring.cpp#include <cstring> #include <iostream> int main() { const char* mainString = "Repeat, repeat, and repeat again"; const char* subString = "repeat"; char* result = strstr(mainString, subString); while (result != nullptr) { std::cout << "Found at: " << result - mainString << std::endl; result = strstr(result + 1, subString); } return 0; }
Here, after finding each occurrence, the pointer is advanced, and
strstr()
is called again to find the next occurrence, demonstrating how to find multiple appearances of a substring.
Conclusion
The strstr()
function is an essential component of the C++ string handling functions, providing a straightforward method to locate substrings within larger strings. This article walked you through its basic usage, along with handling edge cases and advanced scenarios like finding multiple occurrences. Use these techniques to enhance your string processing capabilities in C++, aiding in tasks from basic parsing to complex data processing.
No comments yet.