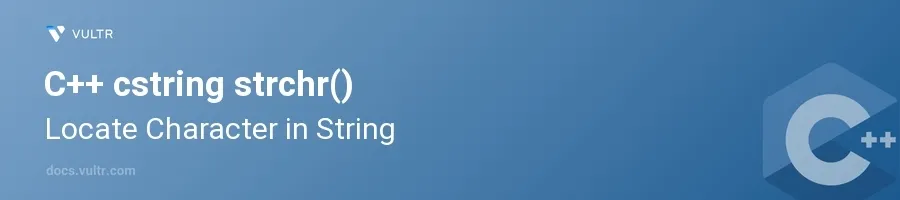
Introduction
The strchr()
function in C++ is a part of the C-style string manipulation functions and is used to locate the first occurrence of a specific character within a string. This function plays a crucial role in parsing and manipulating C-style strings, especially when searching for delimiters or specific characters that decide the flow of logic in string processing tasks.
In this article, you will learn how to effectively use the strchr()
function to search for characters within C-style strings. Explore how this function can help in various string-related operations, enhancing efficiency and control in string manipulation.
Using strchr() to Locate Characters
Basic Usage of strchr()
Include the
<cstring>
library wherestrchr()
function is defined.Prepare a C-style string.
Call
strchr()
to find a character in the string.cpp#include <iostream> #include <cstring> int main() { const char* str = "Hello, World!"; const char* result = strchr(str, 'W'); if (result != nullptr) { std::cout << "Character 'W' found at position: " << result - str << std::endl; } else { std::cout << "Character not found." << std::endl; } return 0; }
This code searches for the character 'W' in the string "Hello, World!". If found, it prints the position of 'W'; otherwise, it informs that the character is not present.
Handling Characters That Are Not Found
Understand that
strchr()
returns a null pointer if the character is not found.Implement proper checks to handle this scenario efficiently.
cpp#include <iostream> #include <cstring> int main() { const char* str = "Example string"; char search_char = 'z'; const char* result = strchr(str, search_char); if (result != nullptr) { std::cout << "Character '" << search_char << "' found at position: " << result - str << std::endl; } else { std::cout << "Character '" << search_char << "' not found." << std::endl; } return 0; }
In this example, the character 'z' is searched in the string "Example string". The check for null pointer ensures that the program can handle cases where the character does not exist in the string.
Using strchr() in a Loop for Repeated Searches
Use
strchr()
in a loop to find multiple occurrences of a character.Update the search start position after each find to continue the search.
cpp#include <iostream> #include <cstring> int main() { const char* str = "Find all the 'a' in this sentence."; const char target = 'a'; const char* result = strchr(str, target); while (result != nullptr) { std::cout << "Found '" << target << "' at position: " << result - str << std::endl; result = strchr(result + 1, target); } return 0; }
This code will loop through the string and print the position of each 'a' found. The subsequent searches start just after the previously found character by incrementing the pointer.
Conclusion
The strchr()
function in C++ is a vital tool for string manipulation, particularly when you need to locate specific characters within a string. By mastering its use, you enhance your ability to manage C-style strings effectively. Implementing these techniques ensures robust and efficient text processing in your C++ applications, whether it involves simple searches or complex parsing operations.
No comments yet.