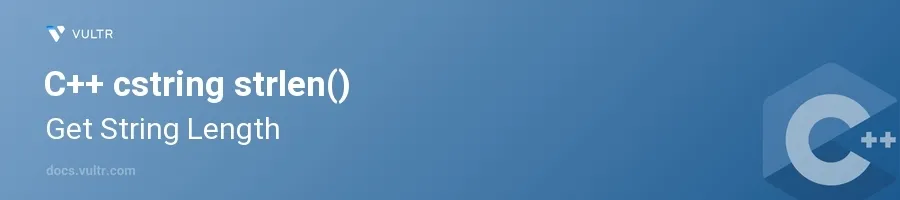
Introduction
The strlen()
function in C++ is vital for obtaining the length of a string. Its primary utility comes from determining the number of characters in a C-style string (character array) before the terminating null character. This function forms an essential part of the <cstring>
header, commonly used in situations where string handling is necessary without the overhead of C++ string classes.
In this article, you will learn how to effectively utilize the strlen()
function to retrieve the length of C-style strings in various programming scenarios. Explore its straightforward application and understand some nuances related to different types of char arrays.
Utilizing strlen() in C++
Basic Usage of strlen()
Include the
<cstring>
library in your C++ program to accessstrlen()
.Declare a C-style string initialized with a constant character array.
Use
strlen()
to obtain the length of the string.cpp#include <iostream> #include <cstring> int main() { const char str[] = "Hello, World!"; size_t len = strlen(str); std::cout << "Length of the string: " << len << std::endl; }
This code snippet shows how to measure the length of "Hello, World!", which returns 13, as it does not include the null character that terminates the string.
Handling Strings with Null Characters
Recognize that
strlen()
stops counting when it encounters the first null character (\0
).Create a C-style string that includes a null character within the string.
Apply
strlen()
to see how it calculates the length up to the first null character.cpp#include <iostream> #include <cstring> int main() { const char str[] = "Hello\0World"; size_t len = strlen(str); std::cout << "Length of the string before first NULL character: " << len << std::endl; }
In this example,
strlen()
calculates the length as 5 (from 'H' to the first '\0'). This behavior is crucial to note when handling data that may contain null characters unexpectedly.
Using strlen() with Dynamically Allocated Strings
Dynamically allocate a C-style string using a pointer.
Ensure proper memory allocation and handling to avoid memory leaks.
Use
strlen()
to determine the string's length and then free the allocated memory.cpp#include <iostream> #include <cstring> int main() { char* str = new char[20]; strcpy(str, "Dynamic String"); size_t len = strlen(str); std::cout << "Length of the dynamic string: " << len << std::endl; delete[] str; }
Here, the program allocates memory dynamically, copies "Dynamic String" into it, and then measures its length. Memory is properly cleaned up after use, exemplifying good management practice in dynamic situations.
Conclusion
The strlen()
function in C++ offers a consistent and efficient way to determine the length of a C-style string. Understanding how strlen()
operates, from handling static and dynamically allocated strings to managing strings that contain null characters within them, is crucial. Implement these techniques to ensure your code handles strings correctly and efficiently, while always keeping memory management in focus. Utilize strlen()
to your advantage in project scenarios that involve low-level string manipulation.
No comments yet.