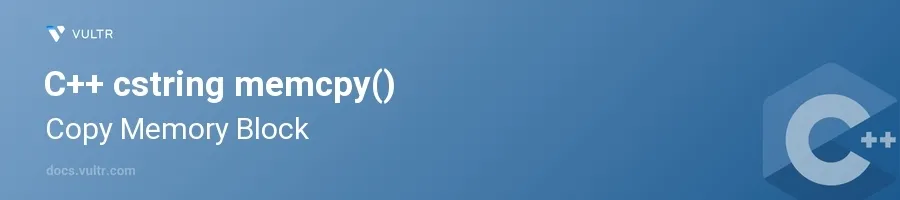
Introduction
The memcpy()
function in C++ is essential for copying blocks of memory from one location to another. This standard library function provides a quick and reliable method for data manipulation in memory, which is especially useful in systems programming, embedded systems, and performance-critical applications where direct memory access is necessary.
In this article, you will learn how to effectively use the memcpy()
function in C++. Explore its parameters, understand its usage through examples, and learn about important considerations to avoid common pitfalls such as overlapping memory areas.
Understanding memcpy() Function
Syntax and Parameters
The memcpy()
function is declared in the cstring
header (also known as string.h
in C) and its prototype is as follows:
void* memcpy(void* dest, const void* src, size_t num);
- Understand the function parameters:
dest
: Pointer to the destination array where the content is to be copied.src
: Pointer to the source of data to be copied.num
: Number of bytes to copy.
Basic Usage Example
Include the
cstring
header in your C++ program.Define source and destination arrays.
Use
memcpy()
to copy data from the source to the destination.cpp#include <iostream> #include <cstring> int main() { char src[] = "Copy this string"; char dest[50]; memcpy(dest, src, strlen(src) + 1); std::cout << "Dest string: " << dest << std::endl; return 0; }
This example copies a string from
src
todest
including the null-terminator (strlen(src) + 1
ensures the null-terminator is copied).
Use Case with Non-Character Data
Recognize that
memcpy()
can work with any data type.Assume working with integer array data.
Copy the integer array using
memcpy()
.cpp#include <iostream> #include <cstring> int main() { int src[] = {1, 2, 3, 4, 5}; int dest[5]; memcpy(dest, src, sizeof(src)); for(int i = 0; i < 5; ++i) std::cout << dest[i] << " "; std::cout << std::endl; return 0; }
Here,
sizeof(src)
is used as thenum
parameter inmemcpy()
which calculates the total bytes occupied by thesrc
array.
Guarding Against Overlapping Memory
- Understand that
memcpy()
should not be used ifsrc
anddest
regions overlap. - Use
memmove()
for overlapping regions instead.
Overlapping Memory Example Using memmove()
Create a char array.
Try to copy a section to an overlapping region within the same array.
Use
memmove()
for safe overlap handling.cpp#include <iostream> #include <cstring> int main() { char data[20] = "Overlap Example"; memmove(data + 7, data, strlen(data) + 1); std::cout << "Data: " << data << std::endl; return 0; }
This code snippet safely copies the string within the same array to an overlapping destination using
memmove()
.
Conclusion
The memcpy()
function in C++ serves as a fundamental tool for efficient memory copying in programming, applicable across various data types. Ensure that you use memcpy()
correctly by avoiding overlapping memory situations. For such cases, prefer memmove()
, which is designed to handle overlaps. Embrace these C-like operations to boost the performance of your C++ applications, especially when dealing with large blocks of data.
No comments yet.