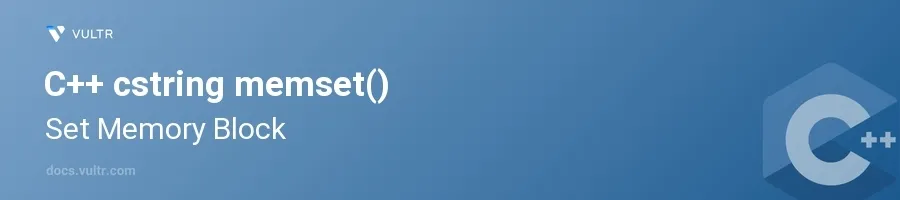
Introduction
The memset()
function in C++ is a powerful utility from the C Standard Library that serves to set a block of memory with a specific value. The memset()
function is available in C++ via the <cstring>
header and is crucial when initializing or resetting large arrays or buffers consistently and quickly within C++ programs.
In this article, you will learn to use the memset()
function to manage memory in your C++ applications efficiently. Discover practical examples illustrating how to initialize different types of data structures, ensuring your programs are not only optimized but also maintain clarity and effectiveness in managing memory.
Understanding C++ memset() Function
Basic Usage of memset() Function in C++
First, include the C standard library header
<cstring>
which contains thememset()
function.Define a buffer or an array you want to initialize.
Use
memset()
to fill this buffer or array.cpp#include <cstring> char buffer[10]; memset(buffer, 0, sizeof(buffer));
In this C++ code snippet, the
memset()
function fills the entirebuffer
with zeros, ensuring all elements are reset.
Using C++ memset() Function to Set Custom Values to Memory Blocks
Determine the custom value to fill the memory block with, ensuring it fits within the type range of the data array.
Apply the
memset()
function, specifying the memory block, the value, and the number of bytes to be set.cppint numbers[5]; memset(numbers, -1, sizeof(numbers));
Here, the integer array
numbers
is filled with the byte pattern of-1
, typically used to initialize all bits to1
for integer types due to how bytes are represented in memory.
Advanced Applications of C++ memset() Function
Initializing Complex Data Structures
Consider the type of the data structure and its alignment and padding in memory.
Regarding data handling, the
memset()
function does not care what data type the memory holds. It treats the memory as raw bytes (unsigned char
) and sets each byte to the value you give.Correctly apply
memset()
to avoid unintended overwrites due to padding.cppstruct MyData { int id; double value; char name[20]; }; MyData data; memset(&data, 0, sizeof(data));
This usage of
memset()
correctly initializes each field in the structureMyData
to zero, including padding and alignment bytes.
Pitfalls and Precautions
Be aware that
memset()
works at the byte level and does not consider higher-level constructs like constructors in C++.Use
memset()
primarily for POD (Plain Old Data) types or arrays of such types.cppstd::string words[3]; memset(words, 0, sizeof(words));
This code is not recommended as
std::string
involves dynamic memory management which is not handled bymemset()
and may lead to undefined behavior or memory corruption.
Conclusion
The memset()
function is a critical tool for memory management in C++ programming, ideal for setting large memory areas quickly and efficiently. It's best suited for simple data or arrays where complex constructors or destructors are not involved. By incorporating memset()
in appropriate scenarios, you can optimize your system's memory utilization and ensure that your arrays and structures are predictably initialized. Use memset()
effectively and apply it judiciously in scenarios involving basic data types to make your programs robust and error-free.
No comments yet.