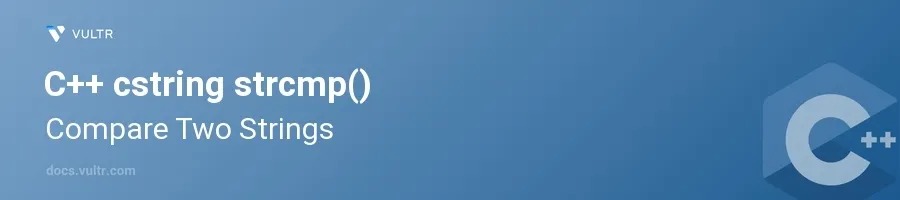
Introduction
The strcmp()
function in C++ is a standard library function that compares two C-style strings (null-terminated). It determines the lexographical order of the strings and is crucial for operations that require string comparison, such as sorting or validating equality. This function is part of the C-string library <cstring>, and it provides an efficient method for evaluating the relationship between two strings.
In this article, you will learn how to use the strcmp()
function to compare two strings. This includes understanding its return values, dealing with typical string comparison scenarios, and handling edge cases effectively.
Understanding strcmp()
Basic Usage and Syntax
Include the <cstring> header in your C++ program to make use of the
strcmp()
function.Understand that
strcmp()
takes two constant character pointers (C-style strings) as arguments and returns an integer.cpp#include <cstring> int main() { const char* str1 = "Hello"; const char* str2 = "World"; int result = strcmp(str1, str2); return 0; }
The
strcmp()
function comparesstr1
andstr2
. The return value stored inresult
will dictate the lexicographical order of the strings, which is discussed next.
Interpretation of Return Values
Analyze the integer returned by
strcmp()
to determine the relationship between the two strings:- A return value of
0
indicates that both strings are equal. - A negative return value suggests that the first string (
str1
) is lexicographically less than the second string (str2
). - A positive return value implies that the first string (
str1
) is lexicographically greater than the second string (str2
).
cppif (result == 0) { std::cout << "Strings are equal." << std::endl; } else if (result < 0) { std::cout << "First string is less than the second string." << std::endl; } else { std::cout << "First string is greater than the second string." << std::endl; }
By evaluating
result
, effectively determine the lexicographical relationship betweenstr1
andstr2
.- A return value of
Common Use Cases of strcmp()
Comparing Similar Strings
Use
strcmp()
to determine if two strings that look similar are actually the same.cppconst char* str1 = "hello"; const char* str2 = "hello"; int result = strcmp(str1, str2);
Since
str1
andstr2
are identical,strcmp()
will return0
.
Case Sensitivity Considerations
Recognize that
strcmp()
is case-sensitive, which affects its comparison outcome.Directly compare two strings with differing cases to see how
strcmp()
behaves.cppconst char* str1 = "Hello"; const char* str2 = "hello"; int result = strcmp(str1, str2);
The comparison will result in a non-zero value, indicating the strings are different due to case sensitivity.
Conclusion
The strcmp()
function in C++ is an essential tool for comparing C-style strings. It offers precise lexographical comparisons and can be used to sort arrays of strings or validate string equality. By understanding and using the examples provided, effectively leverage strcmp()
in your applications to handle string comparisons efficiently and accurately. Whether comparing file paths, user inputs, or any data derived from strings, strcmp()
will provide reliable and consistent results.
No comments yet.