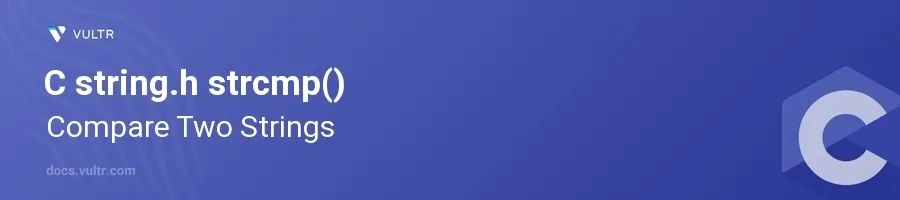
Introduction
In C programming, comparing two strings is a common task that can be performed using the strcmp()
function from the string.h
library. This function is essential for operations like sorting lexicographically or checking string equality, which are foundational in many algorithms and applications that deal with textual data.
In this article, you will learn how to utilize the strcmp()
function to compare two strings. Discover the significance of its return values and how it can be effectively implemented in various scenarios to compare strings accurately.
Understanding strcmp()
Basic Usage of strcmp()
Include the
string.h
library to use thestrcmp()
function.Declare two string variables for comparison.
Call
strcmp()
with the two strings as arguments.c#include <string.h> #include <stdio.h> int main() { char str1[] = "apple"; char str2[] = "apple"; int result = strcmp(str1, str2); printf("Comparison result: %d\n", result); }
This code snippet compares
str1
andstr2
. Since both strings are identical,strcmp()
returns0
.
Analyzing Return Values
Understand that
strcmp()
returns an integer.- It returns
0
if both strings are identical. - A negative value if the first non-matching character in the first string is less than that in the second string.
- A positive value if the first non-matching character in the first string is greater than that in the second string.
- It returns
Extend the program to include different comparisons.
c#include <string.h> #include <stdio.h> int main() { char str1[] = "apple"; char str2[] = "banana"; char str3[] = "apple"; char str4[] = "apricot"; printf("Compare 'apple' and 'banana': %d\n", strcmp(str1, str2)); printf("Compare 'apple' and 'apple': %d\n", strcmp(str1, str3)); printf("Compare 'apple' and 'apricot': %d\n", strcmp(str1, str4)); }
This expanded example shows various comparisons. The results will illustrate how strings are compared lexically based on ASCII values of characters.
Practical Applications of strcmp()
Case Sensitivity in String Comparisons
Note that
strcmp()
is case sensitive.Modify input strings to demonstrate the case sensitivity effect.
c#include <string.h> #include <stdio.h> int main() { char str1[] = "Apple"; char str2[] = "apple"; printf("Case sensitive comparison: %d\n", strcmp(str1, str2)); }
Since 'A' and 'a' have different ASCII values, the function will return a non-zero value, indicating the strings are not identical.
Sorting Strings
Use
strcmp()
for sorting an array of strings.Implement a simple sorting algorithm, such as bubble sort, utilizing
strcmp()
.c#include <string.h> #include <stdio.h> void sortStrings(char arr[][50], int n) { char temp[50]; for (int j=0; j < n-1; j++) { for (int i=j+1; i < n; i++) { if (strcmp(arr[j], arr[i]) > 0) { strcpy(temp, arr[j]); strcpy(arr[j], arr[i]); strcpy(arr[i], temp); } } } } int main() { char arr[][50] = {"banana", "apple", "mango"}; int n = sizeof(arr) / sizeof(arr[0]); sortStrings(arr, n); for(int i = 0; i < n; i++) { printf("%s\n", arr[i]); } }
This function sorts an array of strings in ascending order based on their lexicographical order.
Conclusion
The strcmp()
function in C is a versatile and powerful tool for comparing two strings. Understanding the integer it returns enables the determination of not only the equality but also the lexical order of strings. Implement this function in various scenarios, from simple string equality checks to complex array sorting. By applying the techniques discussed, you enhance the robustness and functionality of your C programs.
No comments yet.