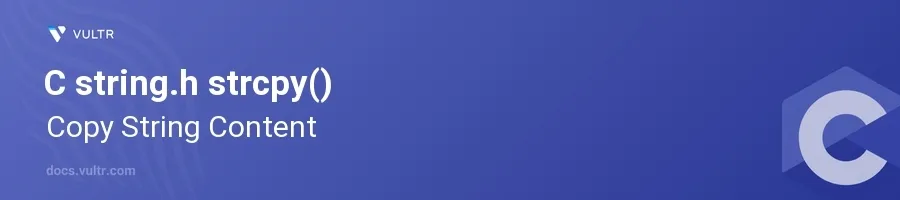
Introduction
The strcpy()
function from the string.h
library in C is a standard library function used to copy the content of one string into another. This function is fundamental in C programming when handling string operations, such as duplicating strings or initializing one string with the content of another.
In this article, you will learn how to effectively utilize the strcpy()
function in C programming. You will explore various scenarios where strcpy()
is applicable, ensuring string copying is done safely and correctly.
Basics of strcpy()
Understanding strcpy() Syntax and Parameters
Review that
strcpy()
takes two parameters: a destination string and a source string.Know that the destination string must have enough space to store the copied content.
cchar source[] = "Hello, World!"; char destination[20]; strcpy(destination, source); printf("Copied String: %s\n", destination);
The
strcpy(destination, source);
line copies the string fromsource
todestination
. The output isCopied String: Hello, World!
, showing that the content was copied successfully.
Potential Risks when Using strcpy()
Be aware that not checking the size of the destination buffer can lead to buffer overflow, a common vulnerability in C programming.
Ensure the destination array is large enough to include the source string and the null terminator,
\0
.cchar source[] = "This is a potentially long string"; char destination[15]; // Dangerously small buffer! strcpy(destination, source); // Unsafe use of strcpy()
This code snippet is unsafe because the
destination
buffer is too small to holdsource
, potentially leading to buffer overflow.
Preventing Buffer Overflow in strcpy()
Using strncpy() for Safer Copy
Consider using
strncpy()
as an alternative tostrcpy()
. It takes an additional parameter – the number of characters to copy, which enhances safety.Use
strncpy()
to copy string content safely by specifying the maximum number of characters to copy.cchar source[] = "Safe copying with strncpy"; char destination[25]; strncpy(destination, source, sizeof(destination) - 1); destination[24] = '\0'; // Make sure it's null-terminated printf("Copied String: %s\n", destination);
This example demonstrates how to safely copy the string to avoid overflow. The explicit null termination ensures the string is properly terminated.
Conclusion
The strcpy()
function in C is a commonly used tool for copying strings, but it requires careful use to avoid buffer overflow issues. By understanding how to use strcpy()
and its safer variant, strncpy()
, you can handle string copying more securely in your C programs. Ensure always to validate that the destination buffer has sufficient space to hold the entire source string, including the null terminator. By following the practices shown, your C programs remain robust and secure from common vulnerabilities associated with string operations.
No comments yet.