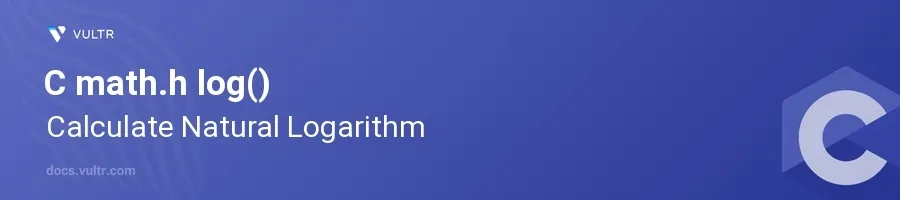
Introduction
The log()
function in C, provided by the math.h
header, calculates the natural logarithm of a given number. Understanding the application of this function is crucial for tasks involving exponential and logarithmic operations, which are common in mathematical computations, finance, and engineering disciplines. Natural logarithms are particularly valuable because they use the base e (approximately 2.718), which has unique properties in calculus and exponential growth models.
In this article, you will learn how to apply the log()
function in various programming contexts using the C programming language. Explore the simple application cases, handling special values, and the consequences of providing invalid input, enhancing your mathematical computation abilities in C.
Using log() to Calculate Natural Logarithms
Basic Usage of log()
Include the
math.h
header file in your program as it contains the prototype forlog()
.Pass a positive double value to
log()
and capture the result.c#include <stdio.h> #include <math.h> int main() { double value = 5.0; double result = log(value); printf("The natural logarithm of %.2f is %.2f\n", value, result); return 0; }
This code snippet calculates the natural logarithm of 5.0. Remember, the input to
log()
must always be a positive number, as logarithm for non-positive numbers is undefined.
Handling Special Values
Be aware that passing zero or negative numbers to
log()
leads to domain errors.Capture special values like
NAN
and-HUGE_VAL
in results to handle errors.c#include <stdio.h> #include <math.h> #include <errno.h> #include <fenv.h> int main() { double zero = 0.0, negative = -1.0; double logZero = log(zero); double logNegative = log(negative); printf("log(0.0) = %.2f, domain error: %s\n", logZero, (errno == EDOM) ? "Yes" : "No"); printf("log(-1.0) = %.2f, domain error: %s\n", logNegative, (errno == EDOM) ? "Yes" : "No"); return 0; }
The output for
log(0.0)
is-inf
, and forlog(-1.0)
, it isnan
. Both indicate inappropriate input as natural logarithms are only positive real numbers.
Conclusion
The log()
function in C is essential for calculating the natural logarithms of positive numbers, a common mathematical operation in many fields. Using log()
from math.h
, you can handle complex exponential and growth formulations with easy and applicable programming constructs. Remember to manage special values and exceptions to maintain robustness in your applications. Fully utilise this function to simplify and streamline the implementation of logarithmic calculations in your software solutions.
No comments yet.