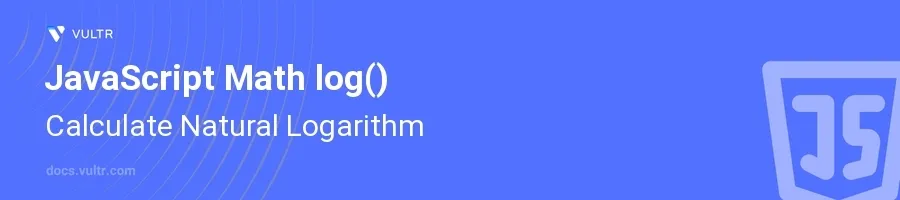
Introduction
The Math.log()
function in JavaScript is used to calculate the natural logarithm (base e
) of a number. This mathematical function is critical in many scientific, statistical, and financial calculations where growth or decay is modeled exponentially. Understanding how to apply Math.log()
effectively can enhance the accuracy and performance of your numerical computations within your JavaScript applications.
In this article, you will learn how to harness the power of the Math.log()
function across several use cases. Explore the practical applications in computing natural logarithms for various types of input values and how it interacts with other mathematical functions to solve complex calculations.
Basic Usage of Math.log()
Calculate Natural Logarithm of a Positive Number
Define a positive number for which you wish to calculate the natural logarithm.
Use
Math.log()
to perform the calculation.javascriptconst number = 10; const naturalLog = Math.log(number); console.log(naturalLog);
This code calculates the natural logarithm of the number 10. The resulting output will display the computed value.
Handling Non-positive Numbers
Understand that
Math.log()
returns NaN if applied to a negative number and negative infinity for zero.Set up conditions to handle these specific cases appropriately.
javascriptconst negativeNumber = -10; const zeroNumber = 0; const logNegative = Math.log(negativeNumber); const logZero = Math.log(zeroNumber); console.log(logNegative); // Outputs NaN console.log(logZero); // Outputs -Infinity
This code demonstrates handling special cases when using natural logarithms. Outputs are NaN for negative numbers and -Infinity for zero.
Working with Other Math Functions
Combine Math.log()
with Math.exp()
to Verify Inverse Properties
Calculate the natural logarithm of a number and then apply the exponential function to confirm the inverse relationship.
Verify that the output approximates the original number.
javascriptconst originalNumber = 5; const logValue = Math.log(originalNumber); const expValue = Math.exp(logValue); console.log(expValue); // Outputs approximately 5
This example first applies
Math.log()
to compute the natural logarithm and thenMath.exp()
to find the exponential value of the logarithm. This process essentially reverses the log operation, demonstrating its inverse property.
Conclusion
Math.log()
in JavaScript serves as a powerful function for calculating the natural logarithm of a number, essential in various mathematical and practical applications. Apply this function effectively in conjunction with other Math
module functions to handle complex numbers and perform accurate calculations. Mastery of Math.log()
enhances the mathematical capabilities of your JavaScript projects and ensures robust handling of exponential and logarithmic data models.
No comments yet.