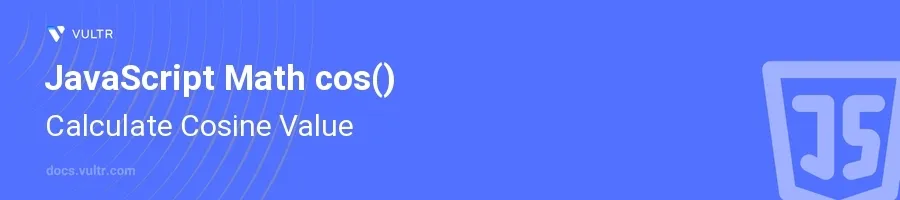
Introduction
The Math.cos()
method in JavaScript is a built-in function that calculates the cosine of a given angle. The angle is provided in radians, making this method very useful in various applications involving trigonometric calculations, such as animations, physics simulations, or any geometry-related problems.
In this article, you will learn how to use the Math.cos()
function effectively. Discover how to convert degrees to radians, calculate cosine values, and explore practical examples to see how this function can be applied in real-world scenarios.
Understanding the Math.cos() Function
Calculate the Cosine of an Angle in Radians
Ensure you understand that
Math.cos()
accepts radians, not degrees.Pass the radian value to the
Math.cos()
function to get the cosine value.javascriptlet radians = Math.PI / 2; // 90 degrees in radians let cosineValue = Math.cos(radians); console.log(cosineValue);
This code snippet calculates the cosine of 90 degrees, converted to radians. The result is approximately
6.123233995736766e-17
, which is effectively0
due to the precision limitations of floating-point arithmetic in JavaScript.
Converting Degrees to Radians
Understand that you often need to convert degrees to radians for trigonometric calculations in JavaScript.
Use the conversion formula: Radians = Degrees * (π / 180).
Apply the conversion before using
Math.cos()
.javascriptlet degrees = 180; let radians = degrees * (Math.PI / 180); let cosineValue = Math.cos(radians); console.log(cosineValue);
Here, the cosine of 180 degrees is calculated. The output is
-1
, as expected for the cosine at this angle.
Practical Applications of Math.cos()
Creating a Simple Harmonic Motion Effect
Use
Math.cos()
to simulate the oscillating motion.Calculate the X position of an object moving in a horizontal sine wave.
javascriptlet time = 0; let amplitude = 100; // maximum distance from the center let period = 60; // number of frames to complete one cycle function updatePosition() { time++; let x = amplitude * Math.cos((2 * Math.PI / period) * time); console.log(x); // Update object position here } setInterval(updatePosition, 16); // approximately 60 FPS
This example calculates the X position of an object as it moves in a simple harmonic motion. It simulates a sine wave pattern, which is useful in animations.
Conclusion
The Math.cos()
function in JavaScript is crucial for performing cosine calculations, particularly in fields involving geometry and trigonometry. By converting degrees to radians and using this function, you can handle various scientific and practical computations. Whether you're developing animations, modeling physical systems, or solving geometry problems, Math.cos()
provides the precision and ease needed to implement trigonometric solutions effectively.
No comments yet.