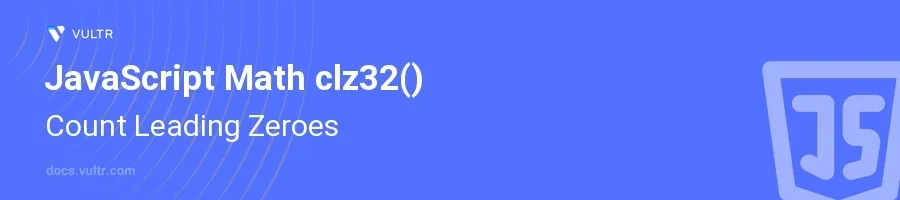
Introduction
The Math.clz32()
method in JavaScript computes the number of leading zeroes in the 32-bit binary representation of a number. This method is particularly useful in systems programming, bitwise operations, and when dealing with low-level data manipulation where efficiency is crucial.
In this article, you will learn how to use the Math.clz32()
function effectively to count leading zeroes in the binary representation of numbers. Explore how this function operates with different types of inputs such as integers, floats, and other data types, and understand its practical applications in various programming contexts.
Understanding Math.clz32()
Usage with Integer Values
Start with a straightforward integer.
Apply
Math.clz32()
and observe the output.javascriptlet count = Math.clz32(1); console.log(count);
This code calculates the leading zero bits in the binary representation of
1
. Since1
in 32-bit binary is00000000000000000000000000000001
, there are 31 leading zeroes.
Behavior with Large Numbers
Use a large integer and apply
Math.clz32()
.javascriptlet count = Math.clz32(1000000); console.log(count);
Given a larger number, the binary representation fills more, reducing the count of leading zeroes. This output will reflect that change.
Handling Zero
Understand that zero is a special case.
javascriptlet count = Math.clz32(0); console.log(count);
Zero (
0
) in 32-bit binary is represented entirely by zeroes, soMath.clz32(0)
returns 32.
Application Scenarios
Use in Bitwise Operations
- Consider situations where bit manipulation is critical, such as graphics programming or hardware interfacing.
- Use
Math.clz32()
to determine padding or alignment needs in data structures.
Optimizing Algorithms
- Use
Math.clz32()
in algorithms where knowing the highest order bit is necessary, such as in radix sorts or binary trees optimizations. - Integrate this method to enhance performances where computations depend on the highest set bit position.
Conclusion
The Math.clz32()
function in JavaScript is invaluable for counting leading zero bits in the 32-bit representation of numbers. From routine tasks like data alignment to critical applications in systems programming, Math.clz32()
aids in developing efficient, performance-optimized software. Implement this method in suitable scenarios to make your JavaScript code more efficient and robust, especially in applications involving low-level data processes and bitwise computation.
No comments yet.