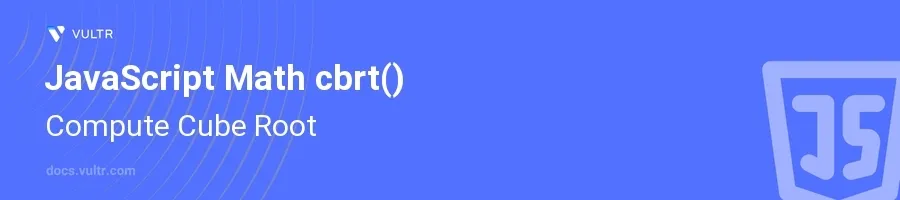
Introduction
The Math.cbrt()
method in JavaScript calculates the cube root of a given number. It is a part of the extensive Math object, which provides utilities for performing complex mathematical operations conveniently. The cbrt()
function is crucial for mathematical computations involving volume and processing geometrical shapes and physics equations where cubing and cube roots are common.
In this article, you will learn how to utilize the Math.cbrt()
method in various scenarios. Discover practical examples that demonstrate its use in calculations, including handling positive numbers, negative numbers, and special numeric values like NaN
and infinity.
Using Math.cbrt() with Numbers
Calculating Cube Roots of Positive Numbers
Provide a positive number as the argument to
Math.cbrt()
.Print the result to observe the cube root.
javascriptvar result = Math.cbrt(27); console.log(result);
This code calculates the cube root of 27, which returns 3 because 3 x 3 x 3 equals 27.
Handling Negative Numbers
Understand that
Math.cbrt()
correctly handles negative inputs.Pass a negative number to see the cube root of a negative value.
javascriptvar negativeResult = Math.cbrt(-8); console.log(negativeResult);
By applying
Math.cbrt()
to -8, the output is -2. This result is accurate as -2 cubed (-2 x -2 x -2) equals -8.
Dealing with Zero and Infinity
Recognize the behavior of
Math.cbrt()
when dealing with zero and infinity.javascriptconsole.log(Math.cbrt(0)); // Outputs 0 console.log(Math.cbrt(Infinity)); // Outputs Infinity console.log(Math.cbrt(-Infinity)); // Outputs -Infinity
In these examples,
Math.cbrt()
demonstrates its ability to manage special numbers effectively, handling both zero and infinity as expected.
Working with Non-Numeric Inputs
Calculating Cube Roots of Non-Numeric Values
Pass non-numeric values to
Math.cbrt()
and handle them appropriately.Check the results when inputs are
NaN
or non-numeric strings.javascriptconsole.log(Math.cbrt('27')); // Converts string to number and calculates console.log(Math.cbrt('a')); // NaN, as 'a' cannot be converted to a number console.log(Math.cbrt(NaN)); // Outputs NaN
These scenarios illustrate that
Math.cbrt()
attempts to convert strings to numbers. However, if the input is not numeric or isNaN
, the result isNaN
.
Conclusion
The Math.cbrt()
function in JavaScript provides a straightforward way to compute cube roots, proving itself versatile across various input types, from positive and negative numbers to special values like zero and infinity. By leveraging the examples and techniques explained, enhance your mathematical operations in JavaScript applications, ensuring robustness and accuracy in computations involving cube roots. Use Math.cbrt()
skillfully to handle a wide range of mathematical tasks efficiently.
No comments yet.