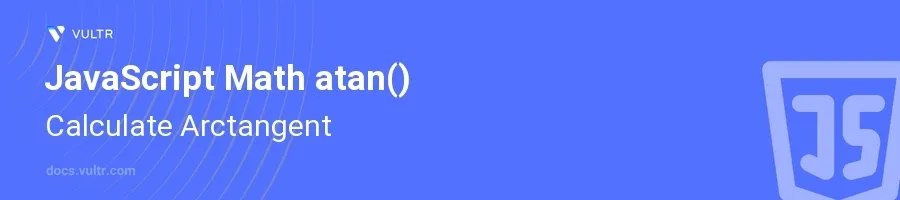
Introduction
The Math.atan()
function in JavaScript is a part of the Math object and is used to calculate the arctangent of a number. This trigonometric function is helpful in scenarios where you need to determine the angle from the X-axis to a point. Typically, this is used in various mathematical, physics, and engineering calculations where angles or gradients are involved.
In this article, you will learn how to effectively utilize the Math.atan()
function in JavaScript. Explore how to calculate angles using this function, understand its return values, and see practical examples that demonstrate its application in real-world scenarios.
Understanding Math.atan()
Basic Usage of atan()
Recognize that
Math.atan()
takes one argument: a number representing the tangent of an angle.The function returns the angle in radians.
javascriptlet tangent = 1; let angleRadians = Math.atan(tangent); console.log(angleRadians);
This example computes the arctangent of 1, which in trigonometry is known to be π/4 radians, approximately 0.785 radians.
Interpreting the Output
Note that the output is in radians, a standard unit of angular measure in many programming languages.
Convert radians to degrees if necessary for clarity or application-specific requirements.
javascriptlet degrees = angleRadians * (180 / Math.PI); console.log(degrees);
Converting the result from the first example into degrees gives approximately 45 degrees, which corresponds to the arctangent of 1.
Practical Applications of Math.atan()
Calculating Slope Angles
Use
Math.atan()
to determine the angle of a slope given its rise and run.This is particularly useful in fields like engineering and physics where understanding the incline angle is necessary.
javascriptlet rise = 3; let run = 3; let slopeAngleRadians = Math.atan(rise / run); let slopeAngleDegrees = slopeAngleRadians * (180 / Math.PI); console.log(`Slope angle: ${slopeAngleDegrees} degrees`);
Here, the slope angle for an equal rise and run results in a 45-degree angle.
Handling Negative Values
Understand that
Math.atan()
can handle negative values, reflecting the cartesian coordinates appropriately.Negative inputs would correspond to angles in the third or fourth quadrants.
javascriptlet negativeTangent = -1; let negativeAngleRadians = Math.atan(negativeTangent); let negativeAngleDegrees = negativeAngleRadians * (180 / Math.PI); console.log(`Angle with negative tangent: ${negativeAngleDegrees} degrees`);
The result indicates a -45 degrees which visually represents an angle pointing down from the X-axis in a standard cartesian plane.
Conclusion
The Math.atan()
function in JavaScript is a fundamental tool for calculating the angle for a given tangent value in radians. Whether you're working on tasks that require precise angle measurements or simply integrating trigonometric calculations within your application, Math.atan()
provides accurate and quick computations. By learning to convert the angles to degrees and understanding the handling of different inputs, you can apply this function in a variety of practical scenarios with confidence.
No comments yet.