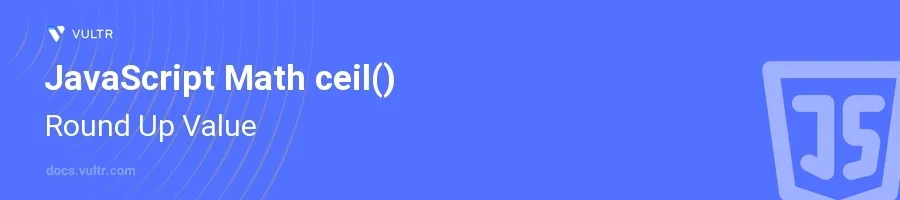
Introduction
The Math.ceil()
function in JavaScript is crucial when you need to round a number up to the nearest integer, regardless of whether the decimal part is small or large. This function ensures that you always get an integer value that is either equal to or greater than the number passed to it, a standard need in various programming contexts involving calculations, especially in financial and graphical computations.
In this article, you will learn how to apply the Math.ceil()
function across different scenarios. Explore how to handle rounding of floating-point numbers and understand the behavior of this function with negative values, zero, and special cases.
Basic Usage of Math.ceil()
Rounding Floating-Point Numbers
Understand that
Math.ceil()
will always round numbers up to the nearest integer.Apply
Math.ceil()
to several floating-point numbers to see its effect.javascriptconsole.log(Math.ceil(4.2)); // Outputs: 5 console.log(Math.ceil(4.9)); // Outputs: 5
These examples demonstrate that
Math.ceil()
rounds both 4.2 and 4.9 up to 5, showing its characteristic of always rounding upwards.
Applying Math.ceil() with Negative Numbers
Note that rounding negative numbers still adheres to rounding up towards zero.
Use
Math.ceil()
with negative floating-point numbers.javascriptconsole.log(Math.ceil(-4.2)); // Outputs: -4 console.log(Math.ceil(-4.9)); // Outputs: -4
Here,
Math.ceil(-4.2)
andMath.ceil(-4.9)
both round up towards zero to -4, instead of moving further away from zero.
Handling Special Cases
Zero and Integer Inputs
Understand that integers and zero, when passed to
Math.ceil()
, remain unchanged because they are already the highest integers less than or equal to themselves.Test
Math.ceil()
with zero and an integer.javascriptconsole.log(Math.ceil(0)); // Outputs: 0 console.log(Math.ceil(5)); // Outputs: 5
In these cases, zero and integer five remain unchanged as there is no decimal part to consider.
Using Math.ceil() with NaN, Infinity, and Other Special Values
Recognize that
Math.ceil()
behaves predictably with special values likeNaN
andInfinity
.Examine the output of
Math.ceil()
when used withNaN
, positiveInfinity
, and negativeInfinity
.javascriptconsole.log(Math.ceil(NaN)); // Outputs: NaN console.log(Math.ceil(Infinity)); // Outputs: Infinity console.log(Math.ceil(-Infinity)); // Outputs: -Infinity
The function retains
NaN
andInfinity
values as they are, showing howMath.ceil()
correctly handles these special cases without altering them.
Conclusion
The Math.ceil()
function in JavaScript is a versatile tool that helps round any given number up to the nearest integer. Its straightforward implementation and predictable behavior with floating-point numbers, negative values, zeros, integers, and even special values make it a valuable asset in your JavaScript toolkit. By mastering Math.ceil()
, you enhance your capability to handle various mathematical transformations efficiently in your projects, ensuring precise and reliable outcomes in calculations.
No comments yet.