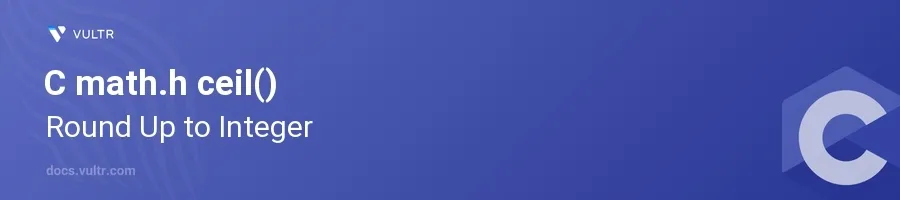
Introduction
In C programming, rounding numbers is a common requirement, whether for ensuring proper allocation of resources, setting bounds, or in computation where precision matters but integer values are needed. The math.h
library provides several functions to handle such tasks, with ceil()
being specifically used to round up floating point numbers to the nearest integer.
In this article, you will learn how to effectively use the ceil()
function to round up floating-point numbers to their nearest integer in C. Explore various examples that demonstrate the function's use in different scenarios, expanding its application beyond basic rounding to include practical real-world programming use cases.
Understanding the ceil() Function
Basic Usage of ceil()
Include the
math.h
header in your C program.Pass a floating-point number to the
ceil()
function.c#include <stdio.h> #include <math.h> int main() { double num = 9.2; double result = ceil(num); printf("Ceiling of %.1f is %.0f\n", num, result); return 0; }
This example rounds the number
9.2
up to the next integer, which is10
. Theceil()
function effectively ignores the decimal part and bumps the whole number up.
Handling Negative Values
Recognize how
ceil()
treats negative numbers.Apply
ceil()
to a negative floating point number.c#include <stdio.h> #include <math.h> int main() { double num = -3.7; double result = ceil(num); printf("Ceiling of %.1f is %.0f\n", num, result); return 0; }
With
ceil()
, even negative numbers are rounded up towards zero. In this case,-3.7
rounds up to-3
.
Real-world Scenario: Calculating Items Needed
Calculate the number of packages needed when each package can hold a certain number of items.
Use
ceil()
to ensure that even a fraction of a package requirement results in an additional full package.c#include <stdio.h> #include <math.h> int main() { double items = 250; double capacity = 24; // items per package double packages_needed = ceil(items / capacity); printf("Need %.0f packages to hold %lf items\n", packages_needed, items); return 0; }
This calculation uses
ceil()
to round up the result ofitems / capacity
. It ensures every item has a package, even if the last package is not completely full.
Conclusion
The ceil()
function in the math.h
library is an invaluable tool in C for rounding up floating-point numbers to the nearest integer. It guarantees upward rounding regardless of whether the number is positive or negative, providing a straightforward and predictable behavior. This function proves particularly useful in scenarios where you need to calculate elements like resource allocation, determining array sizes, and more, making it indispensable in many programming tasks. By integrating ceil()
into projects, you assure that calculations conform to the needs of various applications requiring strict rounding rules.
No comments yet.