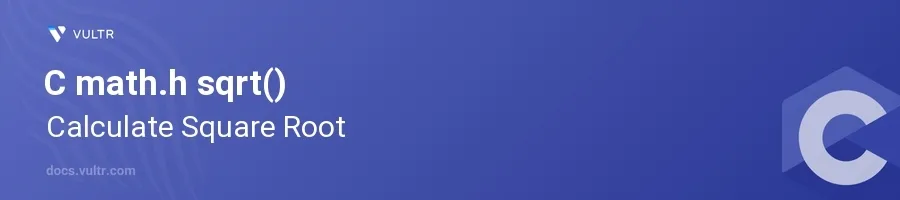
Introduction
The sqrt()
function from the C standard library, defined in the math.h
header, compute the square root of a given number. This function is essential when performing mathematical computations where determining the root of a number is required, such as in engineering, physics simulations, or when applying certain algorithms in data analysis.
In this article, you will learn how to effectively use the sqrt()
function in the C programming language. Discover how to include it in your programs, understand its input requirements, and see practical examples that demonstrate its usage.
Understanding sqrt() Function
Basic Usage of sqrt()
Include the
math.h
header in your C program sincesqrt()
is part of this library.Call the
sqrt()
function with a number of which you want to calculate the square root.#include <stdio.h> #include <math.h> int main() { double number = 9.0; double result = sqrt(number); printf("The square root of %.1f is %.1f\n", number, result); return 0; }
Here,
sqrt(number)
calculates the square root of the variablenumber
. The program will outputThe square root of 9.0 is 3.0
, demonstrating the calculation's result.
Handling Advanced Scenarios
Understand that
sqrt()
accepts non-negative numbers. Passing a negative value leads to a domain error.In situations with negative inputs, consider how to handle the error or transform the input.
#include <stdio.h> #include <math.h> #include <errno.h> #include <fenv.h> int main() { double number = -9.0; double result = sqrt(number); if (errno == EDOM) { printf("Error: Negative input provided to sqrt().\n"); } else { printf("The square root of %.1f is %.1f\n", number, result); } return 0; }
This code checks for a domain error which occurs if a negative number is used. It handles the error by notifying the user instead of attempting to display an invalid result.
Using sqrt() in Complex Calculations
Integrate
sqrt()
in formulae requiring square root calculations.Use it to calculate dynamic values where the input may vary during runtime.
#include <stdio.h> #include <math.h> int main() { for (int i = 0; i <= 10; i++) { double area = i * i * M_PI; // Area of a circle with radius i double circleRadius = sqrt(area / M_PI); printf("Radius calculated from area %.2f is %.2f\n", area, circleRadius); } return 0; }
This example calculates the area of circles with radii from 0 to 10 and then uses
sqrt()
to find the radius back from each area, emphasizing the function's use in reverse calculations.
Conclusion
Utilizing the sqrt()
function in the C programming language allows for the efficient calculation of square roots in various scientific, engineering, and data processing applications. By incorporating the examples provided, you enhance your ability to handle different computation types — from simple square root calculations to more complex scenarios involving error handling and dynamic calculations. Always ensure that the use cases for sqrt()
align with its capabilities, mainly that the function only accepts non-negative values unless specifically handled.
No comments yet.