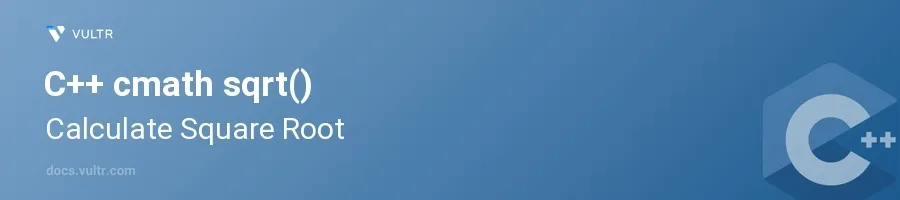
Introduction
The sqrt()
function in C++ is a standard library function used to compute the square root of a number. This function is part of the <cmath>
library and provides a straightforward way to obtain the square root of both floating-point and integral values. Understanding how to leverage this function can enhance the precision and efficiency of your numerical computations in C++ applications.
In this article, you will learn how to use the sqrt()
function to calculate square roots in C++. You will explore practical examples that demonstrate its application in various computational scenarios.
Using sqrt() in Basic Computations
Calculating the Square Root of a Number
Include the
<cmath>
library in your C++ program.Declare and initialize a variable with the value for which the square root is to be calculated.
Use the
sqrt()
function to compute the square root.Output the result using
std::cout
.cpp#include <iostream> #include <cmath> int main() { double number = 25.0; double squareRoot = sqrt(number); std::cout << "The square root of " << number << " is " << squareRoot << std::endl; }
In this code,
sqrt()
calculates the square root of25.0
, which is5.0
. The result is then printed to the console.
Handling Negative Numbers
Realize that the square root of a negative number is not a real number.
Check if the number is negative before attempting to compute its square root.
Display an appropriate message if the number is negative.
cpp#include <iostream> #include <cmath> int main() { double number = -16.0; if (number < 0) { std::cout << "Square root of negative numbers is not defined in the real number system." << std::endl; } else { double squareRoot = sqrt(number); std::cout << "The square root of " << number << " is " << squareRoot << std::endl; } }
This snippet checks if the number is negative before using
sqrt()
. Since-16.0
is negative, it outputs a message rather than attempting the calculation.
Advanced Uses of sqrt()
Using sqrt() in Geometric Calculations
Apply
sqrt()
to calculate the hypotenuse in a right triangle using the Pythagorean theorem.Initialize two variables for the lengths of the perpendicular sides.
Compute the hypotenuse and print the result.
cpp#include <iostream> #include <cmath> int main() { double side1 = 3.0; double side2 = 4.0; double hypotenuse = sqrt(side1*side1 + side2*side2); std::cout << "The length of the hypotenuse is: " << hypotenuse << std::endl; }
By squaring
side1
andside2
, adding these values, and then taking the square root of the result, this code calculates the hypotenuse of a right triangle, outputting5.0
.
Conclusion
The sqrt()
function in C++ is an essential tool for handling square root calculations effectively. Whether performing basic arithmetic operations or engaging in more complex geometric computations, understanding how to use sqrt()
expands your ability to solve a variety of mathematical problems. By incorporating the function correctly, you improve the accuracy and performance of your C++ applications.
No comments yet.