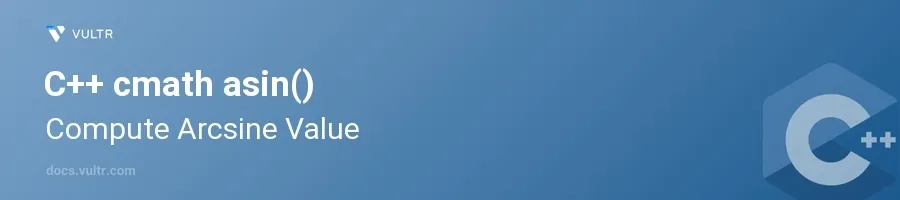
Introduction
The C++ asin()
function is a part of the <cmath>
library and is used to calculate the arcsine (inverse sine) of a given value. The function returns the angle in radians whose sine is the specified value, making it indispensable in fields such as geometry, physics, and engineering where trigonometry plays a crucial role.
In this article, you will learn how to use the asin()
function to compute arcsine values in various contexts. Understand the input restrictions, explore its usage with different data types, and see practical examples that demonstrate how to integrate this function into C++ programs for effective problem-solving.
Understanding asin() Function
Basic Usage
Include the
<cmath>
library in your C++ program.Provide a floating-point number between
-1
and1
as input to theasin()
function.cpp#include <iostream> #include <cmath> int main() { double result = asin(0.5); std::cout << "The arcsine of 0.5 is: " << result << " radians" << std::endl; }
This code calculates the arcsine of
0.5
, which is approximately0.523599
radians.
Handling Input Outside the Valid Range
Validate inputs to ensure they are within the accepted range.
Process using conditional statements to avoid runtime errors.
cpp#include <iostream> #include <cmath> int main() { double x = 1.5; if(x >= -1 && x <= 1) { double result = asin(x); std::cout << "The arcsine of " << x << " is: " << result << " radians" << std::endl; } else { std::cout << "Error: Input should be between -1 and 1." << std::endl; } }
In this snippet,
asin()
is conditionally called only if the input valuex
is within the range-1
to1
. This prevents undefined behavior and runtime errors.
Impact of Precision and Data Types
Experiment with different data types to observe the precision of the
asin()
function's output.Compare the results of
asin()
when usingfloat
versusdouble
.cpp#include <iostream> #include <cmath> int main() { float x = 0.5f; double y = 0.5; float result_float = asin(x); double result_double = asin(y); std::cout << "Using float: " << result_float << " radians" << std::endl; std::cout << "Using double: " << result_double << " radians" << std::endl; }
This example demonstrates the difference in precision between using a
float
and adouble
with theasin()
function. Typically,double
provides higher precision thanfloat
, which can be critical in applications requiring high accuracy.
Conclusion
The asin()
function in C++ serves as a robust tool for calculating the arcsine of a number. It plays a vital role in applications involving trigonometric computations. By ensuring inputs are within the valid range and understanding how the function behaves with different data types, you can effectively utilize asin()
to solve problems requiring inverse sine calculations. Utilize this function to boost the mathematical capabilities of your next C++ project, ensuring precise and reliable results.
No comments yet.