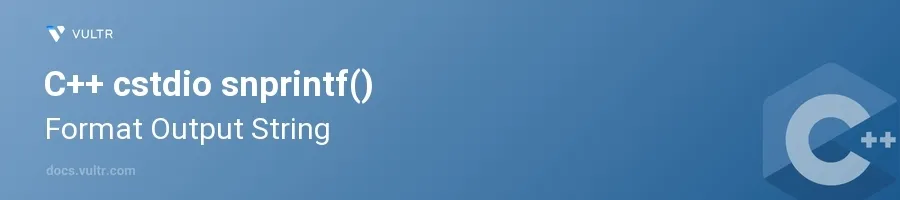
Introduction
The snprintf()
function in C++ is an essential tool for formatting output strings. Derived from the C standard library, it provides robust control over string formatting, allowing developers to create a formatted string by specifying a format and a set of arguments. This function is especially useful for ensuring that the output conforms to a specific pattern while avoiding buffer overflow, which is a common issue with its counterpart, sprintf()
.
In this article, you will learn how to skillfully use the snprintf()
function to format and output strings in C++. The discussion includes how to handle basic string formatting, set width and precision, and deal with different data types, enhancing both the security and flexibility of your code.
Basic Usage of snprintf()
Formatting a Simple String
Define a character array to hold the formatted output.
Use
snprintf()
to write formatted data into the buffer.cpp#include <cstdio> char buffer[100]; int length = snprintf(buffer, sizeof(buffer), "Hello, %s!", "World"); printf("Formatted String: %s\n", buffer);
This code formats a greeting into the
buffer
. The%s
placeholder is replaced with"World"
, and the resulting output is stored inbuffer
.
Handling Integer Formatting
Prepare variables for numeric data.
Format an integer using
snprintf()
.cppchar num_buffer[50]; int num = 2023; snprintf(num_buffer, sizeof(num_buffer), "Year: %d", num); printf("Output: %s\n", num_buffer);
Here, the
%d
format specifier is used for formatting the integer value stored innum
.
Setting Width and Precision
Specifying Minimum Field Width
Set a fixed width for the numeric output.
Use
snprintf()
to align the number in a field of a specified width.cppchar width_buffer[50]; int temperature = 25; snprintf(width_buffer, sizeof(width_buffer), "Temperature: %10d", temperature); printf("Formatted Output: '%s'\n", width_buffer);
This formats
temperature
within a field of width 10, right-aligned by default.
Formatting Floating-Point Numbers
Control the number of digits after the decimal.
Format a
double
variable to include a specific precision.cppchar float_buffer[50]; double pi = 3.14159265; snprintf(float_buffer, sizeof(float_buffer), "Pi: %.2f", pi); printf("Pi value: %s\n", float_buffer);
Using
%.2f
, thesnprintf()
function formatspi
to two decimal places.
Handling Various Data Types
Formatting a Hexadecimal Value
Understand the format specifier for hexadecimals.
Use
%x
or%X
to format an integer as hexadecimal.cppchar hex_buffer[50]; int some_number = 255; snprintf(hex_buffer, sizeof(hex_buffer), "Hex: %X", some_number); printf("Formatted Hex: %s\n", hex_buffer);
This code demonstrates formatting an integer (
some_number
) as a hexadecimal string in uppercase.
Conclusion
Mastering the snprintf()
function in C++ significantly bolsters your capability to create formatted strings safely and effectively. With its ability to prevent buffer overflows and dynamically specify formatting through various specifiers, it serves as a crucial tool for both simple and advanced string handling tasks. By incorporating the techniques discussed, you ensure your C++ applications handle string outputs proficiently, maintaining both readability and data integrity across diverse programming scenarios.
No comments yet.