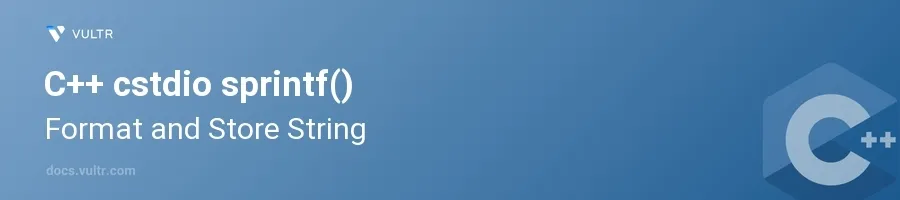
Introduction
The sprintf()
function in C++ is part of the cstdio library, and it serves a crucial role in formatted string manipulation. Programmers use this function to create formatted strings and store them in a buffer specified as an argument. This functionality is central to generating text that needs to adhere to a specific format, which is common in file outputs, logs, or any situation where formatted data presentation is crucial.
In this article, you will learn how to effectively utilize the sprintf()
function for string formatting in C++. Explore various examples that demonstrate its flexibility and power in real-world coding scenarios.
Understanding sprintf()
Basic Syntax and Usage
Recognize the function prototype:
C++int sprintf(char *str, const char *format, ...);
Analyze each component:
str
: A pointer to the buffer where the formatted string is stored.format
: A format string that specifies how to format the input values....
: A list of arguments that need to be formatted according to the format string.
Format Specifiers
- Understand common format specifiers:
%d
for integers%f
for floating point values%c
for a single character%s
for strings
Example: Formatting an Integer
Use
sprintf()
to format and store an integer.C++char buffer[50]; int num = 10; sprintf(buffer, "The number is %d", num);
This code sets
buffer
to the string "The number is 10".sprintf()
processes the format string and the number, placing the formatted text inbuffer
.
Advanced Usage of sprintf()
Handling Multiple Data Types
Combine integers, strings, and floating-point numbers in a single
sprintf()
call.C++char buffer[100]; char* name = "Alice"; int age = 30; double height = 5.5; sprintf(buffer, "%s is %d years old and %.1f feet tall.", name, age, height);
This outputs "Alice is 30 years old and 5.5 feet tall." into
buffer
.
Precision and Padding
Format a floating-point number with set precision.
C++char buffer[50]; double pi = 3.14159; sprintf(buffer, "Formatted Pi: %.3f", pi);
The string "Formatted Pi: 3.142" is now stored in
buffer
, demonstrating controlled precision.
Conditional Formatting
Use logic to conditionally format a string based on content.
C++char buffer[100]; int sales = 150; sprintf(buffer, "Sales %s target.", (sales > 100 ? "exceeded" : "did not meet"));
Depending on the value of
sales
,buffer
will either contain "Sales exceeded target." or "Sales did not meet target."
Conclusion
The sprintf()
function in C++ offers a versatile method for formatting strings in numerous ways, catering to a wide range of scenarios from simple text formatting to complex conditional statements. By mastering sprintf()
, writing and managing formatted strings becomes more efficient and streamlined, enhancing the readability and maintainability of your code. Utilize the techniques discussed to handle various formatting tasks skillfully within your C++ projects.
No comments yet.