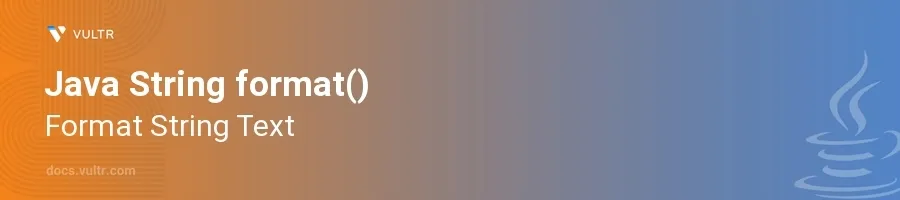
Introduction
The format()
method in Java is a versatile tool provided in the String
class to format strings. It allows you to construct formatted strings with placeholders, making it highly useful for creating dynamic content where variable values need to be embedded within text. Whether for aligning text for console output or preparing strings that include numbers, dates, and other data with specific formatting, format()
is essential.
In this article, you will learn how to effectively utilize the format()
method in various Java programming scenarios. Explore how this method can help manage controlled output and discover its benefits in real-world applications.
Understanding the Basics of String.format()
A Simple Example of Formatting Text
Start by constructing a basic format string with placeholders.
Use the
String.format()
method to replace placeholders with actual values.javaString name = "John"; String message = String.format("Hello, %s!", name); System.out.println(message);
In this example,
%s
is a placeholder for a string. The value "John" replaces%s
, resulting in "Hello, John!" being printed to the console.
Formatting Numbers
Realize that formatting numbers allows for better control over decimal places and alignment.
Employ
String.format()
to format floating-point numbers.javadouble price = 19.99; String formattedPrice = String.format("Price: $%.2f", price); System.out.println(formattedPrice);
This code formats the number
19.99
to two decimal places, producing the outputPrice: $19.99
.
Including Multiple Variables in a Formatted String
Combine multiple placeholders within a single string to include various data types.
Execute the
format()
method to create a sentence with these values.javaint quantity = 5; double total = price * quantity; String summary = String.format("Quantity: %d, Total: $%.2f", quantity, total); System.out.println(summary);
Here,
%d
is used for an integer and%f
for a floating-point number, with both placeholders replaced byquantity
andtotal
respectively, resulting in a formatted summary.
Advanced Formatting Options
Formatting Dates
Explore using
String.format()
to include date information.Format the date in a friendly or specific pattern.
javaimport java.util.Date; String formattedDate = String.format("Current Date: %tF", new Date()); System.out.println(formattedDate);
%tF
stands for a complete date format (ISO 8601), resulting in output similar toCurrent Date: 2023-09-15
.
Conditional Formatting with Ternary Operators
Utilize conditional logic inside the
format()
method to handle different scenarios dynamically.Construct a formatted string that adapts to the value of a variable.
javaint stock = 3; String stockMessage = String.format("Stock Status: %s", stock > 0 ? "Available" : "Out of stock"); System.out.println(stockMessage);
This example checks the
stock
value to determine the message. Ifstock
is greater than 0, "Available" is displayed; otherwise, "Out of stock".
Aligning and Padding Strings
Enhance formatted strings with padding and alignment options to improve text readability.
Use adjusted placeholders for left or right alignment along with specifying field width.
javaString leftAligned = String.format("|%-10s|", "Left"); String rightAligned = String.format("|%10s|", "Right"); System.out.println(leftAligned); System.out.println(rightAligned);
Here,
%10s
pads the string on the left for a total width of 10 characters, resulting in a right-aligned output, and%-10s
does the opposite.
Conclusion
The format()
method in Java's String class furnishes you with extensive capabilities for string manipulation, especially for formatting output data. By mastering String.format()
, you can control text alignment, incorporate multiple variables into strings, and customize numeric and date/time formatting effortlessly. Implement these techniques in your Java applications to produce more readable, professional outputs that effectively communicate data states and values to users. Employ the examples discussed to start integrating flexible and dynamic text formatting in your Java projects.
No comments yet.