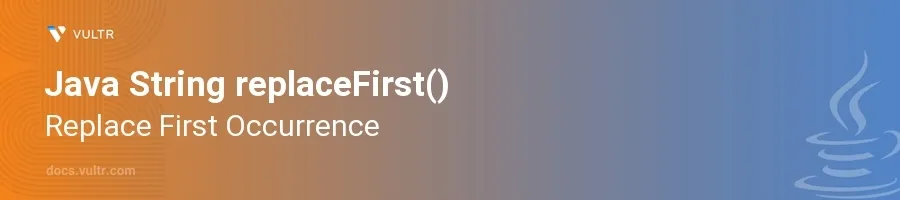
Introduction
Java's replaceFirst()
method in the String
class is a powerful tool for manipulating strings by replacing the first occurrence of a specified substring with another. This method is highly useful when you need precise control over the text transformation, especially in data processing or user input sanitization.
In this article, you will learn how to effectively use the replaceFirst()
method to modify strings. Understand how to target specific patterns, including regular expressions, to replace the first instance that matches the criteria, enhancing your capabilities in string manipulation within Java applications.
Understanding the replaceFirst() Method
Basic Usage of replaceFirst()
Recognize that
replaceFirst()
is a method intended to replace the first occurrence of a specified substring with a replacement in the invoking string.To utilize the method, reference it from a string object and pass two parameters: a regular expression that specifies the pattern to replace, and the replacement string.
javaString sentence = "cats, cats, and more cats!"; String modifiedSentence = sentence.replaceFirst("cats", "dogs"); System.out.println(modifiedSentence);
This code replaces the first instance of "cats" with "dogs". The output will be "dogs, cats, and more cats!".
Handling Regular Expressions
Realize that the first parameter of
replaceFirst()
takes a regular expression, offering versatile matching capabilities.Experiment with regular expressions to match different patterns or characters.
javaString info = "Email: user@example.com"; String updatedInfo = info.replaceFirst("[aeiou]", "*"); System.out.println(updatedInfo);
In this code snippet, the first vowel in the string is replaced by an asterisk. The output would be "Em*il: user@example.com".
Using replaceFirst() with Special Characters
Understand that special characters in regular expressions, such as
.
(dot),(
(parenthesis), and*
(asterisk), have specific meanings and need to be escaped properly.Use double backslashes (
\\
) to escape special characters in the regular expression string.javaString filename = "document.doc"; String newFilename = filename.replaceFirst("\\.doc", ".txt"); System.out.println(newFilename);
Here, the
.doc
extension is replaced with.txt
. With\\.doc
, the.
is treated literally rather than as a wildcard character, which represents any character in regular expressions. The output is "document.txt".
Practical Example: Normalizing Phone Numbers
Leverage
replaceFirst()
to standardize or normalize input data, such as phone numbers.Use regular expressions to selectively replace parts of the string.
javaString phone = "+1 (555) 123-4567"; String normalizedPhone = phone.replaceFirst("\\+1 ", ""); System.out.println(normalizedPhone);
The code removes the country code from the phone number if it starts with
+1
. The output would be "(555) 123-4567".
Conclusion
The replaceFirst()
function in Java's String class is a versatile method for altering strings by replacing the first occurrence of a pattern defined by a regular expression. Its ability to handle complex replacements using regular expressions makes it indispensable for tasks requiring precise string manipulation. Whether you're replacing substrings, escaping special characters, or normalizing data formats, replaceFirst()
enhances the flexibility and functionality of your Java applications. By mastering this method, you ensure that string operations in your projects are robust and maintain high-quality standards.
No comments yet.