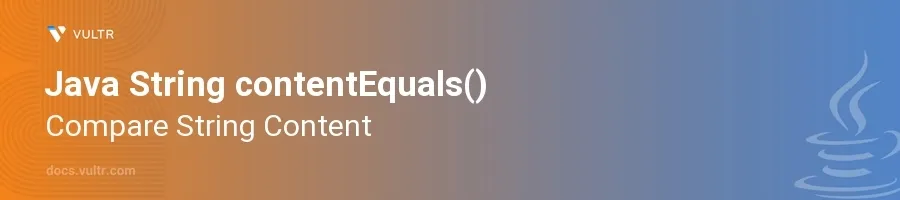
Introduction
The contentEquals()
method in Java is crucial for comparing the content of strings, ensuring an accurate and case-sensitive comparison. This method is particularly useful when the aim is to verify if two different string representations hold the identical sequence of characters, regardless of the object type they originate from, such as StringBuffer
or StringBuilder
.
In this article, you will learn how to use the contentEquals()
method to compare string content effectively in Java. Explore practical examples that demonstrate the method's usage with strings, StringBuffer
, and StringBuilder
. Acquire the skills to deploy this method in various programming scenarios, ensuring precise text comparisons.
Understanding contentEquals()
Method
contentEquals()
is a method provided by the String
class in Java that compares the content of the string against the specified CharSequence
or StringBuffer
. Unlike the equals()
method which only checks against another String
, contentEquals()
allows for more flexible and diverse comparisons.
Here’s a breakdown of how it works:
- The method is invoked from a
String
object. - It accepts either a
CharSequence
orStringBuffer
as an argument. - It returns a boolean value indicating whether the contents are identical.
Consider the following points when using contentEquals()
:
- It compares data character by character.
- It's sensitive to character casing, as are most string comparison tools in Java.
Practical Examples of Using contentEquals()
Comparing String with StringBuffer
Create a
String
and aStringBuffer
with the same contents.Use
contentEquals()
to compare them.javaString str1 = "HelloWorld"; StringBuffer strBuffer = new StringBuffer("HelloWorld"); boolean result = str1.contentEquals(strBuffer); System.out.println(result);
In this code,
str1
is aString
object andstrBuffer
is aStringBuffer
object containing the same text.contentEquals()
compares them, returningtrue
because their contents match exactly.
Comparing Strings with Different Cases
Initialize two
String
objects, one in upper case and the other in lower case.Compare them using
contentEquals()
.javaString str1 = "helloworld"; String str2 = "HELLOWORLD"; boolean result = str1.contentEquals(str2); System.out.println(result);
Here, even though
str1
andstr2
represent the same letters,contentEquals()
evaluates tofalse
due to the case difference.
Use with StringBuilder
Illustrate the flexibility of
contentEquals()
by comparing aString
with aStringBuilder
.Ensure both hold the identical sequence of characters.
javaString str1 = "Java"; StringBuilder strBuilder = new StringBuilder("Java"); boolean result = str1.contentEquals(strBuilder); System.out.println(result);
This demonstrates that
contentEquals()
successfully comparesString
objects againstStringBuilder
instances, here yieldingtrue
because both contain the same sequence "Java".
Handling Nulls
Understand that attempting to use
contentEquals()
with anull
argument triggers aNullPointerException
.Always ensure the argument is not
null
before comparing.javaString str1 = "test"; StringBuffer emptyBuffer = null; try { boolean result = str1.contentEquals(emptyBuffer); System.out.println(result); } catch (NullPointerException e) { System.out.println("Cannot compare to null."); }
This snippet will catch and handle the
NullPointerException
, outputting a warning message instead of crashing the program.
Conclusion
The contentEquals()
method in Java is an incredibly useful tool for doing precise, case-sensitive comparisons between a String
and various CharSequence
implementations such as StringBuffer
and StringBuilder
. As you integrate this method into your applications, always check for null values to avoid NullPointerExceptions
. With the discussed scenarios and examples, harness this method to ensure accurate and efficient text comparisons in your development works. By mastering contentEquals()
, optimize data consistency checks and validation processes throughout your codebase.
No comments yet.