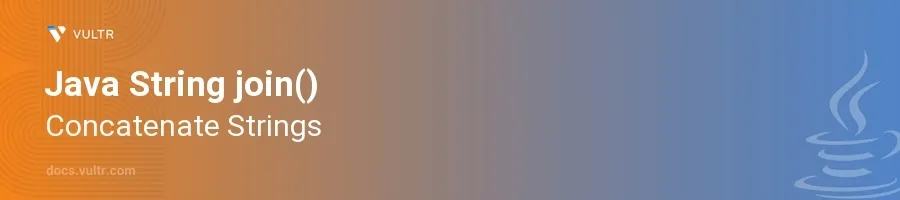
Introduction
The join()
method in Java is a static method in the String
class that concatenates any number of strings together with a specified delimiter. It is particularly useful when you need to build a single string out of multiple substrings, adding a specific separator between each part. This method simplifies the process of string manipulation in Java, especially when dealing with collections or arrays of data that need to be presented as a single string.
In this article, you will learn how to utilize the join()
method to concatenate strings efficiently in Java. Explore various practical scenarios where join()
can enhance code readability and performance, and look at examples that demonstrate using this method with arrays, lists, and handling edge cases.
Utilizing the join() Method
Basic Usage of join()
Understand the signature of the method.
Use the
join()
method to concatenate strings with a comma separator.javaString result = String.join(", ", "Apple", "Banana", "Cherry"); System.out.println(result);
This code combines the strings "Apple", "Banana", and "Cherry" into a single string, separated by commas and spaces. The output will be "Apple, Banana, Cherry".
Concatenating Elements of a List
Collect a list of strings.
Apply the
join()
method to concatenate these strings.javaList<String> fruits = Arrays.asList("Apple", "Banana", "Cherry"); String result = String.join(" - ", fruits); System.out.println(result);
Here, the list
fruits
is converted into a string where each element is separated by " - ". The resulting output is "Apple - Banana - Cherry".
Handling Arrays
Define an array of strings.
Use the
join()
method to concatenate the array’s elements.javaString[] colors = {"Red", "Green", "Blue"}; String result = String.join(" | ", colors); System.out.println(result);
In this snippet, the
join()
method concatenates the elements of thecolors
array, using " | " as the delimiter. The output for this will be "Red | Green | Blue".
Dealing with Null Values and Edge Cases
Check what happens when null values are in the array or list.
Attempt to join strings, including null values.
javaString[] items = {null, "Toaster", null, "Microwave"}; String result = String.join(", ", items); System.out.println(result);
When the
join()
method encountersnull
values in the items array, it treats them as "null" strings in the output, resulting in "null, Toaster, null, Microwave".
Conclusion
The String.join()
method in Java provides a powerful and easy way to concatenate multiple strings with a specified delimiter. Whether you're working with lists, arrays, or any collection of string elements, the join()
method offers a straightforward approach to generate a single, unified string. Employing this method in practical scenarios, such as data formatting or creating compound messages, can greatly enhance the efficiency and readability of your Java programs. Utilize these techniques to ensure your string manipulation processes are both efficient and clear.
No comments yet.