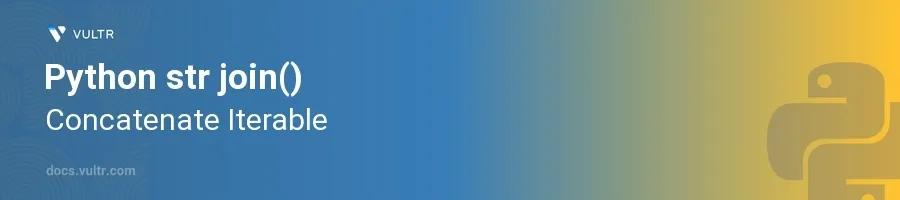
Introduction
The join()
method in Python is a string method that combines an iterable's elements into a single string, separated by a specified separator. This method is often used to concatenate strings efficiently, making it a vital tool in data processing where combining text elements is needed.
In this article, you will learn how to use the str.join()
method to concatenate various types of iterables, such as lists, tuples, and sets. Discover how to apply this function in different scenarios to handle and manipulate string data effectively.
Basic Usage of str.join()
Concatenating List Elements
Start by creating a list of string elements.
Choose a separator string that will be used to join the elements.
Use the
join()
method to concatenate the list's elements into a single string.pythonmy_list = ['apple', 'banana', 'cherry'] separator = ', ' result = separator.join(my_list) print(result)
This code concatenates the elements of
my_list
into a single string, separated by a comma and a space. The output will be"apple, banana, cherry"
.
Handling Various Data Types
Ensure all elements in the iterable are strings. The
join()
method only works with strings, so any non-string data must be converted first.Convert non-string elements to strings within an iteration or using a list comprehension.
Concatenate the modified iterable.
pythonmy_data = [10, 20, 30] result = ', '.join(str(num) for num in my_data) print(result)
Here, each integer in
my_data
is converted to a string usingstr()
, and then these string representations are combined into"10, 20, 30"
.
Advanced Usage with Different Iterables
Using join() with Tuples
Recognize that tuples can be used similarly to lists with
join()
.Store the data in a tuple.
Concatenate the tuple's string elements.
pythonmy_tuple = ('Python', 'Java', 'C++') result = ' - '.join(my_tuple) print(result)
This will output the string
"Python - Java - C++"
, using a hyphen as the separator.
Working with Sets
Understand that using sets with
join()
can lead to unpredictable order, as sets do not maintain order.Define a set with string elements.
Concatenate these using a chosen separator.
pythonmy_set = {'Table', 'Chair', 'Lamp'} result = '; '.join(my_set) print(result)
The output order of the items can vary each time the code is executed because sets do not preserve element order.
Handling Edge Cases
Dealing with Empty Iterables
Test the behavior when the iterable is empty.
Observe that joining an empty iterable results in an empty string.
pythonempty = [] result = ','.join(empty) print('Result:', result) # Output will be 'Result: '
An empty string is printed as there are no elements to join.
Including Separator Logic
Consider how the choice of separator impacts the readability or format of the final string output.
Use conditional logic if varying separators are needed based on the content or length of the iterable.
pythonconditions = ['Rain', 'Sunshine', 'Snow'] result = ' or '.join(condition if condition != 'Snow' else 'cold ' + condition for condition in conditions) print(result)
This example modifies the separator and the elements themselves based on specific conditions, resulting in the string
"Rain or Sunshine or cold Snow"
.
Conclusion
Utilizing the str.join()
method in Python offers a versatile and efficient approach to concatenating strings from an iterable. Whether working with lists, tuples, or even sets, this method facilitates a straightforward way to combine strings effectively. By mastering str.join()
, enhance code readability and maintainability while handling string concatenation tasks with ease. From processing data to generating formatted outputs, this technique proves invaluable in various programming scenarios.
No comments yet.