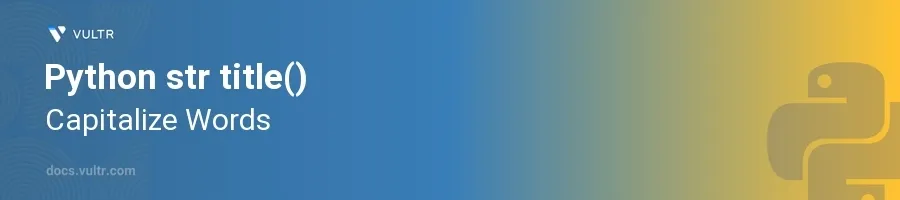
Introduction
The title()
method in Python's string class is a straightforward yet powerful tool for formatting text. It alters a string to ensure that the first letter of every word is capitalized while converting all other letters to lowercase. This function is especially useful for formatting human names, titles in documents, and any other texts where proper capitalization of each word is necessary.
In this article, you will learn how to leverage the title()
method to capitalize words efficiently in Python. You will explore various examples that demonstrate its usefulness and behavior with different types of strings, including those with punctuation and non-standard characters.
Understanding the title() Method
The title()
method is used for string objects and does not require any arguments. It processes the input string and applies title casing, which involves capitalizing the first letter of each word and converting all other letters to lowercase.
Basic Usage of title()
Start with a simple string.
Apply the
title()
method.pythonsimple_string = "hello world" titled_string = simple_string.title() print(titled_string)
This code snippet converts
hello world
toHello World
, capitalizing the first letter of each word.
Title Casing Complex Strings
Use strings that contain apostrophes and hyphens to see how
title()
handles these.Examine the output.
pythoncomplex_string = "o'reilly auto parts" titled_complex_string = complex_string.title() print(titled_complex_string)
In the example above, the word "O'Reilly" might not capitalize as expected because
title()
treats the apostrophe as a word boundary.
Handling Special Cases
While title()
is highly effective, it has limitations, especially with words containing non-standard characters like apostrophes or when dealing with possessive forms. Here's how to address these more complex scenarios.
Issues with Apostrophes and Hyphens
Acknowledge that
title()
might not always produce desired capitalizations with special characters.Consider using a more sophisticated function or regular expressions for more accurate title casing.
pythonimport re def title_case(text): return re.sub(r"[A-Za-z]+('[A-Za-z]+)?", lambda mo: mo.group(0).capitalize(), text) custom_titled_string = title_case("i'm enjoying the star-spangled banner") print(custom_titled_string)
This function uses a regular expression to more precisely identify words, correctly handling complex cases like "I'm" and "Star-Spangled".
Title Casing Non-Standard Characters
Understand the limitations with languages that have non-Latin characters or special grammatical rules.
Employ locale-aware methods if precise capitalization is required for internationalized applications.
python# Python does not natively handle locale-based transformations in title casing. # You would typically need an external library or additional logic specific to each locale.
The comment indicates the need for specialized handling for non-standard characters, which is beyond the scope of Python's built-in methods.
Conclusion
The title()
method in Python provides a simple and efficient way to capitalize the first letter of each word in a string, making it an essential tool for text processing tasks. While it excels in straightforward scenarios, handling more complex strings might require custom solutions, especially when dealing with punctuation or international characters. By understanding its basic functionality and limitations, you can effectively incorporate title()
into your Python applications for various text formatting needs, ensuring the text appears polished and professional. Use this method to transform any textual data, keeping in mind the nuances that might affect the outcome.
No comments yet.