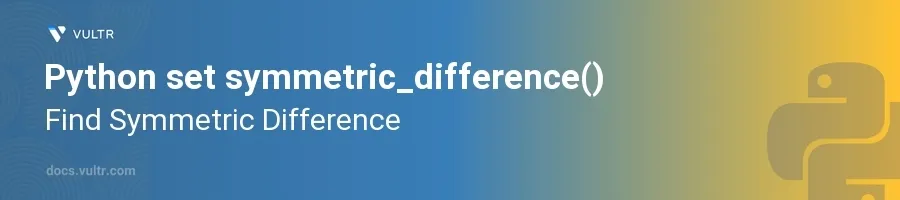
Introduction
The symmetric_difference()
method in Python, associated with sets, is crucial for finding the symmetric difference between two sets. This means it returns a new set containing all items from both sets, excluding the items present in both. This method is quite useful in mathematical and data analysis applications where understanding the differences between datasets is key.
In this article, you will learn how to harness the symmetric_difference()
method in various scenarios to effectively manage and analyze data. Explore practical examples that demonstrate the versatility of this function for performing set operations in Python.
Understanding Symmetric Difference
Basic Use of symmetric_difference()
Define two sets with some common and some unique elements.
Apply the
symmetric_difference()
method to find elements unique to each set.pythonset1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} result = set1.symmetric_difference(set2) print(result)
This code results in a new set
{1, 2, 5, 6}
, excluding the common elements{3, 4}
.
Symmetric Difference with More than Two Sets
Understand that
symmetric_difference()
only operates on two sets at a time.Chain multiple
symmetric_difference()
calls to handle more than two sets.pythonset1 = {1, 2, 3} set2 = {2, 3, 4} set3 = {4, 5, 1} result = set1.symmetric_difference(set2).symmetric_difference(set3) print(result)
This approach sequentially finds the symmetric difference first between
set1
andset2
, and then between the result andset3
, yielding the set{5}
.
Using the ^
Operator
Recognize that the
^
operator is an alternative to thesymmetric_difference()
method.Use the
^
operator between two sets to achieve the same result.pythonset1 = {1, 2, 3} set2 = {3, 4, 5} result = set1 ^ set2 print(result)
This snippet also produces the set
{1, 2, 4, 5}
, demonstrating an alternate but equivalent approach to finding the symmetric difference.
Conclusion
The symmetric_difference()
function is a powerful tool for set operations in Python, enabling clear and effective data analysis through the identification of unique elements in each set. It's straightforward yet versatile and can be used with the traditional method or the shorthand ^
operator. By mastering these techniques, your skills in handling set operations and performing complex data manipulations will greatly improve. The examples provided guide you through different scenarios, ensuring you have the necessary skills to apply these concepts to real-world data analysis tasks.
No comments yet.