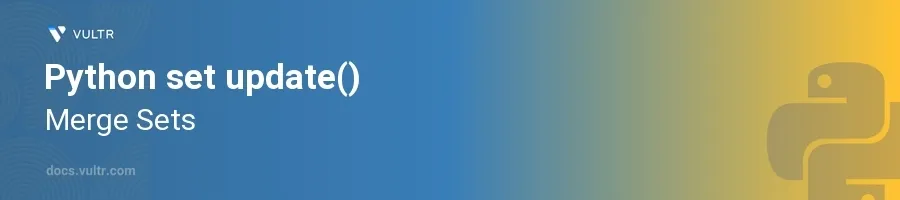
Introduction
The update()
method in Python is an essential tool when working with sets. This method allows the merging of two or more sets, integrating all unique elements from each set involved into a single set without duplication. This functionality is pivotal in scenarios where you need to consolidate data from different sources or when performing set-based operations like unions.
In this article, you will learn how to leverage the update()
method to effectively merge multiple sets. Explore practical examples that illustrate merging sets containing various data types and understand the nuances of this powerful method.
Basics of the update() Method
The update()
method in Python adds elements from a set or any iterable (like lists, tuples, or other sets) to the set on which update()
is called. This method modifies the set in place, meaning it doesn't create a new set but changes the original one.
Add Elements of Another Set
Start with two sets of integer values.
Use the
update()
method to add elements of the second set to the first one.pythonset1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} set1.update(set2) print(set1)
In this example,
set1
originally contains the elements{1, 2, 3, 4}
. After using theupdate()
method withset2
which contains{3, 4, 5, 6}
,set1
becomes{1, 2, 3, 4, 5, 6}
. Note that the duplicates are not added again, showcasing a typical set behavior of unique elements.
Merging Multiple Iterables
Initialize a set with some integer values.
Use the
update()
method to merge this set with multiple iterables, like another set and a list.pythonset1 = {1, 2, 3} set2 = {4, 5} list1 = [5, 6, 7] set1.update(set2, list1) print(set1)
Here,
set1
is initially{1, 2, 3}
. After theupdate()
call withset2
({4, 5}
) andlist1
([5, 6, 7]
), all unique elements from these iterables are added toset1
, resulting in{1, 2, 3, 4, 5, 6, 7}
.
Advanced Usage of update()
Using update() with Different Data Types
Create a set that already has some string elements.
Merge this set with a list containing a mix of integers, strings, and floating-point numbers.
pythonset1 = {'apple', 'banana'} list1 = [1, 'orange', 2.5] set1.update(list1) print(set1)
This code snippet shows
set1
evolving from{'apple', 'banana'}
to include integers and floats fromlist1
, resulting in{'apple', 1, 2.5, 'banana', 'orange'}
. Theupdate()
method works seamlessly with different data types.
Ensuring Idempotence in Set Updates
Recognize that multiple applications of
update()
with the same iterable does not change the set after the first update.Apply
update()
multiple times with the same list to a set.pythonset1 = {1, 2, 3} list1 = [2, 3, 4] set1.update(list1) print("First update:", set1) set1.update(list1) print("Second update:", set1)
This code reaffirms that once
list1
has been used to updateset1
, further updates with the same list don’t make any changes toset1
. Both print statements output the same result,{1, 2, 3, 4}
.
Conclusion
The update()
method in Python sets is invaluable for merging multiple sets or other iterables into a single set efficiently, maintaining uniqueness of elements without duplications. Equipped with techniques outlined above, you can now handle various practical scenarios involving set manipulations and data merging tasks more effectively. Adapt these approaches in different contexts to streamline data consolidation and manipulation in your programs.
No comments yet.