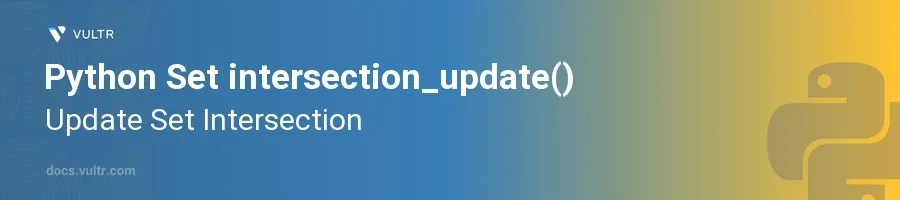
Introduction
The intersection_update()
method in Python is a crucial tool for managing sets when you need to compute and update a set with the intersection of itself and other sets. This method modifies the set on which it's called, allowing you to efficiently handle sets in data processing where set operations are frequent.
In this article, you will learn how to use the intersection_update()
method with various types of data structures. Techniques for handling single and multiple set interactions will be discussed, offering you an understanding of both basic and advanced usage scenarios.
Understanding intersection_update()
Basic Usage
Start with the definition of
intersection_update()
.Apply the method on a simple set example to observe its direct effect.
pythonset1 = {1, 2, 3, 4, 5} set2 = {3, 4, 5, 6, 7} set1.intersection_update(set2) print(set1)
This code sample shows the set
set1
being updated to the intersection ofset1
andset2
. The output will be{3, 4, 5}
, reflecting the common elements between the two sets.
With Multiple Sets
Extend the concept to handling multiple sets at once.
See how
intersection_update()
can be used to modify the original set based on multiple others.pythonset1 = {1, 2, 3, 4, 5} set2 = {3, 4, 5, 6, 7} set3 = {4, 5, 6, 7, 8} set1.intersection_update(set2, set3) print(set1)
By applying
intersection_update()
withset2
andset3
,set1
will now reflect the intersection of all three sets. The result is{4, 5}
as these are the only common elements across the three sets.
Advanced Usage Scenarios
Intersecting with Other Iterables
Explore how
intersection_update()
works with other iterable types, not just sets.Apply the method using a list and a tuple.
pythonset1 = {1, 2, 3, 4, 5} list1 = [4, 5, 6, 7, 8] tuple1 = (5, 6, 7, 8, 9) set1.intersection_update(list1, tuple1) print(set1)
In this example,
set1
is updated to include only elements that are common to the set, the listlist1
, and the tupletuple1
. The resulting set will be{5}
, illustrating howintersection_update()
can work across different iterable types.
Handling Empty Intersections
Understand how
intersection_update()
behaves with non-intersecting sets.Demonstrate the outcome when nothing common exists.
pythonset1 = {1, 2, 3} set2 = {4, 5, 6} set1.intersection_update(set2) print(set1)
Here, since there are no common elements between
set1
andset2
, the result ofset1
after the operation is an empty set{}
.
Conclusion
The intersection_update()
method in Python sets is an invaluable tool for modifying a set to retain only elements found in various intersections. This method provides a direct way to keep only the common elements between the initial set and other sets or iterable types. From managing straightforward two-set intersections to handling complex scenarios involving multiple different iterables, intersection_update()
simplifies many tasks involving set manipulations. Armed with this knowledge, leverage the intersection_update()
method in your Python projects to streamline operations and maintain clean, efficient code.
No comments yet.