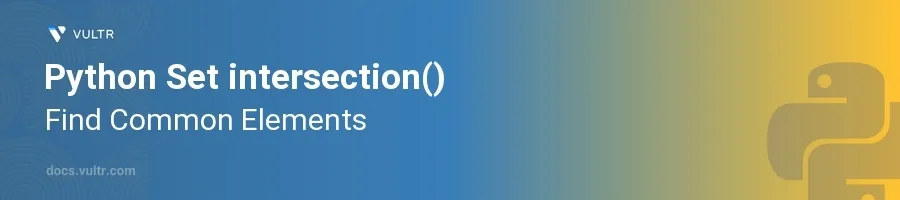
Introduction
Python set intersection, using the intersection()
method, is a powerful tool for computing common elements across multiple sets. This utility is well-suited for operations where you need to identify items that are shared between two or more collections, such as in data analysis, filtering datasets, or when implementing business logic that requires element comparison between groups.
In this article, you will learn how to effectively utilize the intersection()
method with sets in Python. Explore various scenarios showcasing its practical application, ranging from basic to more complex real-world data operations. Discover how this method can simplify tasks and increase efficiency in your Python programming.
Using intersection() with Sets
Find Common Elements in two Sets in python
Create two sets with some overlapping elements.
Apply the
intersection()
method to these sets.pythonset1 = {1, 2, 3, 4, 5} set2 = {4, 5, 6, 7, 8} common_elements = set1.intersection(set2) print(common_elements)
This code snippet finds the common elements between
set1
andset2
. Theintersection()
method analyzes both sets and returns a new set containing the elements that exist in both, which in this case are{4, 5}
.
Multiple Set Intersection
Define more than two sets if the need arises to find a commonality among several collections.
Use
intersection()
to find elements shared by all the sets.pythonset1 = {1, 2, 3, 4, 5} set2 = {3, 4, 5, 6, 7} set3 = {2, 3, 5, 7, 8} result = set1.intersection(set2, set3) print(result)
In this example,
intersection()
identifies{3, 5}
, which are the elements present in all three sets. This capability is crucial for complex data manipulation where multiple dataset overlaps need to be found.
Dynamic Intersection with Iterable Unpacking
Handle cases with a variable number of sets dynamically using iterable unpacking.
Apply the
intersection()
method using the*
operator for unpacking.pythonsets = [ {1, 2, 3}, {2, 3, 4, 5}, {2, 3, 6} ] common = set.intersection(*sets) print(common)
The above code demonstrates finding the intersection of a list of sets dynamically. By unpacking the list of sets into the
intersection()
method, it returns{2, 3}
, the common elements among all contained sets. This approach is very efficient for scenarios where the number of sets is not predetermined.
Advanced Usage of intersection()
Intersection with Comprehensions
Use set comprehensions for more complex conditions within intersection operations.
Combine comprehensions and intersection to filter data based on specific criteria.
pythonset1 = {x for x in range(10) if x % 2 == 0} # Even numbers set2 = {x for x in range(10) if x % 3 == 0} # Multiples of 3 result = set1.intersection(set2) print(result)
This example creates two sets using set comprehensions — one for even numbers and another for multiples of three. Using
intersection()
, it then finds common elements, which are numbers that are both even and multiples of three, outputting{0, 6}
.
Set Overlap in Python
Set overlap in Python refers to identifying common elements between two or more sets. This is done using the intersection() method or the & operator. When two sets share elements, their intersection forms a new set containing only those common elements.
Methods to Find Set Overlap
Using
intersection()
Method.The
intersection()
method returns a set containing elements common to all given sets.pythonset1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} overlap = set1.intersection(set2) print(overlap)
Using
&
Operator.The & operator is a shorthand for finding the intersection between sets.
pythonoverlap = set1 & set2 print(overlap)
Conclusion
The intersection() function in Python sets is instrumental in finding the shared elements between multiple collections efficiently. It provides a clear and concise way to handle common element discovery in a variety of use cases, from simple two-set interactions to complex multi-set configurations. Utilize the techniques discussed to manage and analyze data effectively, ensuring calculations are both accurate and efficient. By embedding these strategies within Python applications, the management and comparison of group datasheets become both streamlined and dependable.
No comments yet.