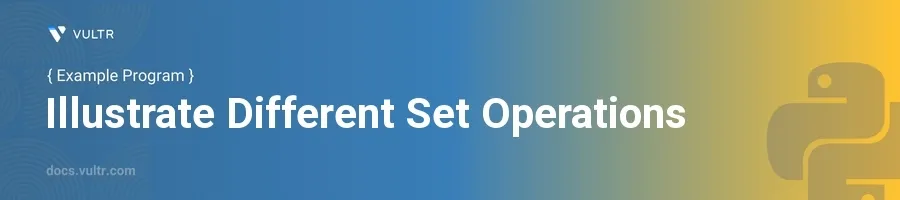
Introduction
A set is a fundamental data structure in Python that represents an unordered collection of unique elements. Sets are particularly useful for carrying out mathematical set operations like unions, intersections, differences, and symmetric differences in an efficient way. Understanding how to manipulate sets and perform these operations can significantly enhance your data handling capabilities in Python.
In this article, you will learn how to perform various set operations in Python through practical examples. Discover how these operations can be applied effectively to solve problems that involve collection-manipulations, such as removing duplicates from data, finding common or distinct items, and more.
Basic Set Operations
Creating and Initializing a Set
Use curly braces
{}
or theset()
constructor to create a set.Initialize a set with unique values.
python# Using curly braces fruits = {'apple', 'banana', 'cherry'} print(fruits) # Using the set constructor numbers = set([1, 2, 3, 2]) print(numbers)
The set
fruits
contains three items, whilenumbers
contains three items, with duplicates removed automatically.
Add and Remove Elements
Use the
add()
method to add an element to a set.Use the
remove()
method to remove a specific element from the set.python# Adding an element fruits.add('orange') print(fruits) # Removing an element fruits.remove('banana') print(fruits)
After adding 'orange', the
fruits
set updates, and then 'banana' is removed from it.
Advanced Set Operations
Union of Sets
Combine elements from two or more sets without duplicates using the
union()
method or the|
operator.pythonset1 = {1, 2, 3} set2 = {3, 4, 5} union_set = set1.union(set2) print(union_set) # Or using the | operator union_set_operator = set1 | set2 print(union_set_operator)
Both snippets produce the set
{1, 2, 3, 4, 5}
, demonstrating the union operation.
Intersection of Sets
Find common elements between sets using the
intersection()
method or the&
operator.pythonintersection_set = set1.intersection(set2) print(intersection_set) # Or using the & operator intersection_set_operator = set1 & set2 print(intersection_set_operator)
The result
{3}
shows the only common element betweenset1
andset2
.
Difference between Sets
Get elements present in one set but not in the other using the
difference()
method or the-
operator.pythondifference_set = set1.difference(set2) print(difference_set) # Or using the - operator difference_set_operator = set1 - set2 print(difference_set_operator)
The output
{1, 2}
consists of elements present inset1
but not inset2
.
Symmetric Difference of Sets
Find elements in exactly one of the sets (not in both) using
symmetric_difference()
or the^
operator.pythonsymmetric_difference_set = set1.symmetric_difference(set2) print(symmetric_difference_set) # Or using the ^ operator symmetric_difference_operator = set1 ^ set2 print(symmetric_difference_operator)
This returns
{1, 2, 4, 5}
showing elements either inset1
orset2
but not in both.
Conclusion
Python's set operations simplify the process of handling collections by offering intuitive methods to perform union, intersection, difference, and symmetric difference among sets. These operations are indispensable for tasks involving data manipulation and are particularly useful in contexts such as data analysis, where handling unique and common elements effectively is essential. By mastering these operations, make data manipulation processes more efficient and your Python programs more robust and cleaner.
No comments yet.