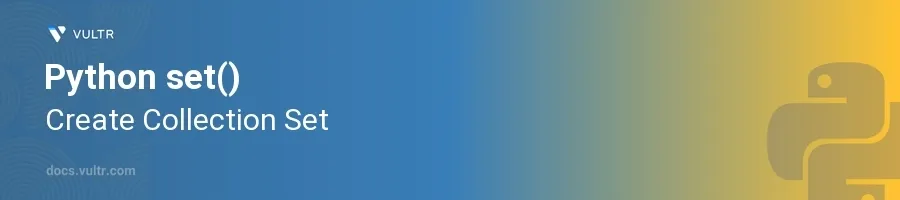
Introduction
The set()
function in Python is instrumental in creating a set, which is an unordered collection of unique elements. This feature can be particularly useful when you need to efficiently remove duplicates from a list, perform set operations like unions, intersections, and differences, or simply when you're looking to ensure that all elements in a collection are distinct.
In this article, you will learn how to use the set()
function to manipulate collections, focusing on creating sets, converting other data types into sets, and applying basic set operations to solve common problems.
Creating Sets in Python
Constructing an Empty Set
Initiate an empty set using the
set()
function without passing any arguments.pythonempty_set = set()
This code snippet creates an empty set named
empty_set
. Notice that the functionset()
is called with no arguments.
Converting Lists to Sets to Remove Duplicates
Prepare a list with duplicate items.
Use the
set()
function to convert this list to a set.pythonmy_list = [1, 2, 2, 3, 4, 4, 4, 5] my_set = set(my_list) print(my_set)
Here,
my_set
will output{1, 2, 3, 4, 5}
. Converting the list to a set removes any duplicate elements.
Using set() With Strings
Understand that converting a string to a set will create a set of its characters.
Apply the
set()
function on a string to see this in action.pythonmy_string = "hello" string_set = set(my_string) print(string_set)
The output will be a set of characters from "hello", such as
{'h', 'e', 'l', 'o'}
, but the exact order may vary as sets are unordered.
Basic Set Operations Using set()
Performing Union Operations
Define two sets of numbers.
Use the
union()
method or the|
operator to combine the sets.pythonset_a = {1, 2, 3} set_b = {3, 4, 5} union_set = set_a | set_b # Alternate: set_a.union(set_b) print(union_set)
This operation combines all unique elements from both sets, resulting in
{1, 2, 3, 4, 5}
.
Finding Set Intersections
Utilize the
intersection()
method or the&
operator to find common elements between two sets.pythonintersection_set = set_a & set_b # Alternate: set_a.intersection(set_b) print(intersection_set)
This code returns
{3}
, which is the common element betweenset_a
andset_b
.
Computing Differences Between Sets
Use the
difference()
method or the-
operator to find elements in one set but not in another.pythondifference_set = set_a - set_b # Alternate: set_a.difference(set_b) print(difference_set)
The output
{1, 2}
shows elements that are inset_a
but not inset_b
.
Conclusion
The set()
function in Python offers a versatile solution for handling collections of unique elements. By transforming lists or other iterables into sets, you efficiently strip out duplicate values and prepare the data for various set-based operations such as unions, intersections, and differences. Implement these techniques in scenarios where data uniqueness is crucial, or when you need to carry out relational data operations. This functionality not only simplifies the code but also optimizes performance by relying on the inherent properties of sets in Python.
No comments yet.