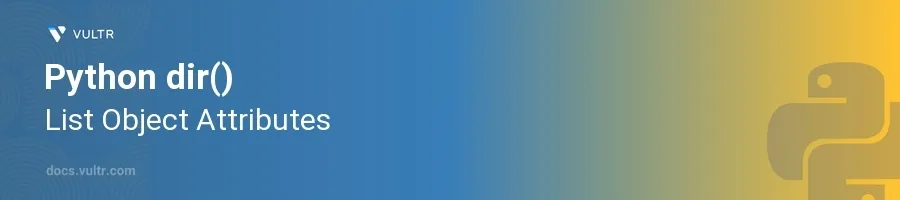
Introduction
The dir()
function in Python provides a convenient way to list all the attributes and methods associated with an object. This function is incredibly useful when exploring new libraries or when you want to understand what operations you can perform with an object without referring to external documentation. It can also be instrumental in debugging, by helping to quickly discover properties or methods.
In this article, you will learn how to utilize the dir()
function to inspect Python objects. Examine how to list attributes for built-in Python data types, user-defined objects, and library modules, providing a broader understanding of Python's functionality and object-oriented programming.
Listing Attributes of Built-In Types
Basic Usage with Integer
Choose an integer variable.
Apply the
dir()
function to reveal its methods.pythonnumber = 42 attributes = dir(number) print(attributes)
This snippet lists the methods associated with an integer in Python. These include methods like
__add__
,__divmod__
, and others that correspond to arithmetic operations and more.
Exploring Attributes of a List
Initialize a list.
Use
dir()
to list its attributes.pythonsample_list = [] list_attributes = dir(sample_list) print(list_attributes)
The output includes list-specific methods such as
append
,extend
,pop
,sort
, and others, showing the operations that can be performed on list objects.
Investigating String Methods
Create a string variable.
Apply
dir()
to discover its methods.pythongreeting = "hello" string_methods = dir(greeting) print(string_methods)
This code lists string methods and attributes, which include formatting methods like
capitalize
,upper
, and manipulation methods likereplace
,split
.
Utilizing dir() with User-Defined Objects
Defining a Simple Class
Define a user-friendly class with several properties and methods.
Instantiate the class and use
dir()
on the instance.pythonclass Vehicle: def __init__(self, make, model): self.make = make self.model = model def display_info(self): return f"Vehicle: {self.make} {self.model}" my_car = Vehicle("Toyota", "Camry") print(dir(my_car))
This example outputs all attributes and methods of the
Vehicle
class, including Python's built-in attributes and the user-defineddisplay_info
method.
Applying dir() on Library Modules
Inspecting a Python Standard Library Module
Import a module, such as
os
.Use
dir()
to list all attributes and methods of the module.pythonimport os os_attributes = dir(os) print(os_attributes)
This command outputs attributes and methods available in the
os
module, providing insights into functionalities like file and directory handling operations.
Conclusion
The dir()
function in Python is an essential tool for introspection, allowing programmers to list all the properties and methods associated with any object. Whether it’s a basic data type, a user-defined class, or a library module, dir()
provides a quick glance at available methods and attributes, facilitating deeper understanding and more effective coding. Recall the examples shown to harness the full potential of this function in your development work, enhancing discovery and debugging processes.
No comments yet.