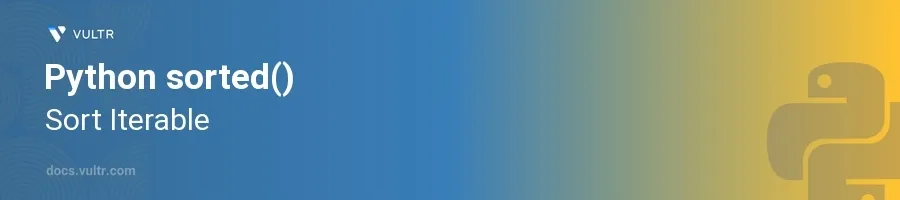
Introduction
The sorted()
function in Python is a built-in utility that arranges elements from any iterable (like lists, tuples, and dictionaries) into a specific order (ascending or descending). This function is integral to data manipulation and preparation in Python, as sorting can often be a preliminary step in data analysis and visualization tasks.
In this article, you will learn how to use the sorted()
function to sort various types of iterables. The discussion will cover basic sorting, customized sorting with key functions, and reverse sorting. Explore how this versatile function can be used to handle different data types and structures effectively.
Sorting Basics
Sort a List
Create a list of integers.
Use the
sorted()
function to sort the list.pythonnumbers = [5, 1, 8, 3, 2] sorted_numbers = sorted(numbers) print(sorted_numbers)
This code snippet sorts the list
numbers
. Thesorted()
function returns a new listsorted_numbers
with elements in ascending order [1, 2, 3, 5, 8].
Sort a String (Alphabetical Order)
Define a string.
Apply
sorted()
to sort the characters of the string.pythongreeting = "hello" sorted_greeting = sorted(greeting) print(sorted_greeting)
Here,
sorted()
converts the string into a list of characters and sorts them alphabetically, resulting in['e', 'h', 'l', 'l', 'o']
.
Customizing Sort with Key Functions
Sort by Length
Create a list of strings.
Sort the list by the length of its elements using
sorted()
with akey
parameter.pythonwords = ["python", "is", "a", "wonderful", "language"] sorted_words = sorted(words, key=len) print(sorted_words)
The
key=len
tellssorted()
to use the length of each element as the criterion for sorting. The output will be['a', 'is', 'python', 'language', 'wonderful']
, sorted based on the number of characters each string contains.
Sort by Custom Function
Define a custom function that influences the sorting order.
Use this function as the key in
sorted()
.pythondef custom_sort(word): return word[-1] # sorts by the last character of the string words = ["banana", "apple", "cherry"] sorted_by_last = sorted(words, key=custom_sort) print(sorted_by_last)
This snippet sorts the
words
list based on the last letter of each word, resulting in['banana', 'apple', 'cherry']
because 'a', 'e', and 'y' are the last letters, respectively.
Reverse Sorting
Sorting in Descending Order
Take any iterable.
Use the
reverse
parameter in thesorted()
function to sort in descending order.pythonnumbers = [5, 1, 8, 3, 2] descending_numbers = sorted(numbers, reverse=True) print(descending_numbers)
Setting
reverse=True
sorts the listnumbers
in descending order, producing[8, 5, 3, 2, 1]
.
Conclusion
The sorted()
function in Python provides a straightforward yet powerful way to order elements from any iterable. Utilizing this function with different types of data, custom key functions, and reverse ordering options allows for flexible data manipulation. By mastering the techniques discussed, enhance the organization and preparation of data in your Python projects, ensuring that you can handle sorting tasks with ease and efficiency.
No comments yet.