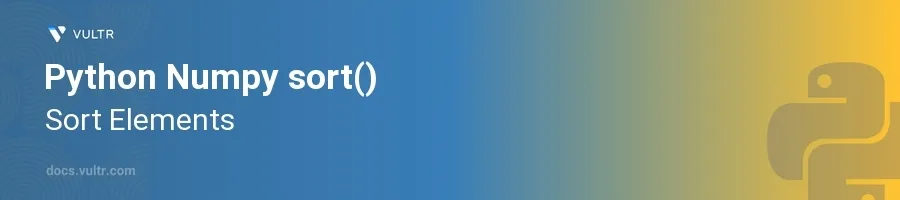
Introduction
The sort()
function in the NumPy library is an essential tool for sorting arrays in Python effectively. Whether it involves sorting numerical data for statistical analysis or arranging items for better visualization, the efficiency of NumPy's sort()
function can significantly streamline the process.
In this article, you will learn how to proficiently use the sort()
function to manage array data. You'll explore how to apply it to various array structures and discover tips for optimal sorting strategies.
Basics of NumPy sort()
Sorting a One-Dimensional Array
Import the NumPy library.
Create a one-dimensional array.
Apply the
sort()
function.pythonimport numpy as np array_1d = np.array([3, 1, 4, 1, 5, 9, 2, 6]) sorted_array = np.sort(array_1d) print(sorted_array)
In this example,
array_1d
is sorted using thenp.sort()
function. The sorted array appears in ascending order, displaying[1, 1, 2, 3, 4, 5, 6, 9]
.
Sorting in Descending Order
Sort the array as usual.
Reverse the array to achieve descending order.
pythondesc_sorted_array = np.sort(array_1d)[::-1] print(desc_sorted_array)
Here, array is first sorted in ascending order and then reversed to get
[9, 6, 5, 4, 3, 2, 1, 1]
in descending order.
Advanced Sorting with NumPy
Sorting a Two-Dimensional Array
Create a two-dimensional array.
Sort along a specified axis.
pythonarray_2d = np.array([[12, 15], [10, 1]]) sorted_array_2d = np.sort(array_2d, axis=0) print(sorted_array_2d)
The code sorts
array_2d
along each column (axis=0), reshuffling the rows to maintain order along columns, resulting in[[10, 1], [12, 15]]
.
Using Different Sorting Algorithms
Understand that NumPy supports various sorting algorithms such as 'quicksort', 'mergesort', and 'heapsort'.
Choose an algorithm to apply.
pythonarray_qs = np.array([3, 1, 4, 1, 5, 9, 2, 6]) sorted_array_qs = np.sort(array_qs, kind='quicksort') print(sorted_array_qs)
This example specifies 'quicksort' as the sorting mechanism, which is usually the default. Other algorithms can be used depending on the efficiency and complexity requirements.
Sorting with Structured Data
Construct a structured NumPy array.
Sort the array based on one of the fields.
pythondtype = [('name', 'S10'), ('age', int)] values = [(b'Alice', 31), (b'Bob', 20), (b'Cathy', 22), (b'Dan', 25)] structured_array = np.array(values, dtype=dtype) sorted_structured_array = np.sort(structured_array, order='age') print(sorted_structured_array)
In the above, the array is sorted by 'age'. This technique is particularly useful when dealing with data that has multiple fields (such as databases or CSV files).
Conclusion
The sort()
function from NumPy in Python provides a robust and efficient method for organizing arrays, whether they're simple, multidimensional, or structured. By mastering the various functionalities and options provided by this function, manipulate large datasets with ease, improving both performance and readability of data handling projects. Harness the full potential of array sorting to optimize data processing tasks in Python applications.
No comments yet.