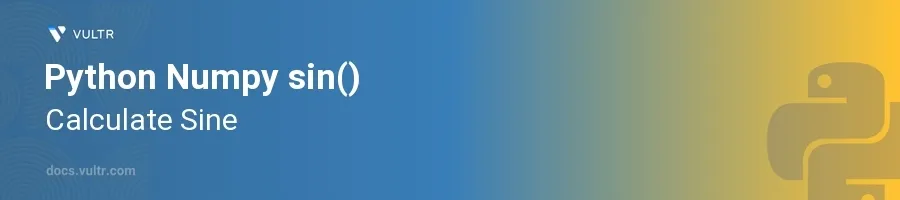
Introduction
The sin()
function from the NumPy library in Python provides a convenient way to compute the sine of various numbers, which is particularly useful in fields like engineering, physics, and data analysis where trigonometric functions are frequently required. NumPy, being a staple for numerical computations in Python, offers this function to handle not only scalars but also arrays of any size, efficiently and effortlessly.
In this article, you will learn how to effectively utilize the sin()
function to calculate the sine of different types and scales of data. Explore handling single values, arrays, and even applying the function over a range of data to generate sine waves or perform vectorized operations for complex calculations.
Using sin() with Single Values
Calculate Sine of a Single Number
First, ensure that NumPy is imported into your Python environment.
Use the
sin()
function to compute the sine of a specific angle provided in radians.pythonimport numpy as np angle_radians = np.pi / 2 # 90 degrees sine_value = np.sin(angle_radians) print(sine_value)
The code above calculates the sine of 90 degrees, converted to radians as
np.pi / 2
. The expected output is1.0
, as the sine of 90 degrees is 1.
Handle Edge Cases
Consider scenarios like calculating the sine of zero, which is expectedly 0.
Experiment with extreme values to understand how
sin()
behaves with them.pythonzero_sine = np.sin(0) extreme_sine = np.sin(np.pi) # Sine of 180 degrees print("Sine of 0 radians:", zero_sine) print("Sine of pi radians:", extreme_sine)
In this snippet,
sin(0)
rightfully results in0.0
andsin(np.pi)
results in a value close to0.0
due to the periodic nature of the sine function.
Using sin() with Arrays
Calculate Sine for a List of Angles
Create a NumPy array of angles in radians.
Apply the
sin()
function across the entire array and print the results.pythonangles = np.array([0, np.pi/2, np.pi, 3*np.pi/2]) sine_values = np.sin(angles) print(sine_values)
This example demonstrates computing the sine of multiple angles at once, outputting the results as an array of sine values corresponding to the angles
[0, π/2, π, 3π/2]
.
Generate a Sine Wave
Use NumPy to create a smoothly spaced array of angles.
Compute the sine values to simulate a sine wave.
pythonx = np.linspace(0, 2*np.pi, 100) # 100 points from 0 to 2π y = np.sin(x)
The array
x
contains 100 values ranging from0
to2*np.pi
, andy
is an array of sine values. This data can be used to plot a sine wave using graphing libraries such as Matplotlib.
Conclusion
The sin()
function in NumPy is a highly effective tool for computing the sine of various data forms in Python. From single scalar values to complex array-based calculations, this function facilitates an array of mathematical and engineering tasks. By employing the sin()
function, leverage the power of NumPy's vectorized operations to enhance the performance and readability of your trigonometric calculations. Apply these techniques to not only compute simple sine values but also generate complex waveforms and patterns efficiently.
No comments yet.