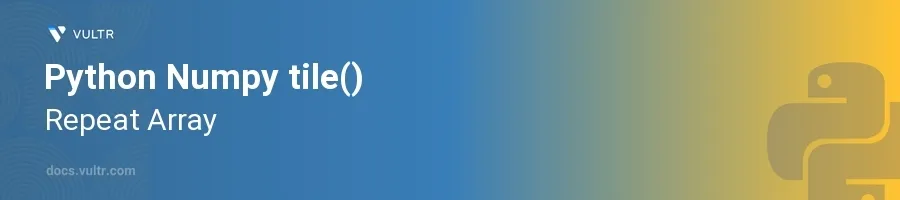
Introduction
The tile()
function from the Numpy library is an essential tool for replicating arrays across multiple dimensions. It's incredibly useful for mathematical and scientific computations where creating large, repeated patterns of data is necessary. Users particularly value this function for its ability to expand the data without the need for cumbersome loops.
In this article, you will learn how to leverage the tile()
function to repeat arrays efficiently. Explore various examples to understand how you can apply this method to both one-dimensional and multi-dimensional arrays, enhancing your array manipulation capabilities in Python.
Basic Usage of Numpy tile()
Repeating a One-dimensional Array
Start by importing the Numpy library.
Create a simple one-dimensional array.
Use the
tile()
function to repeat the array.pythonimport numpy as np # Create a one-dimensional array a = np.array([1, 2, 3]) # Repeat the array 3 times repeated_array = np.tile(a, 3) print(repeated_array)
This code snippet demonstrates repeating the array
[1, 2, 3]
three times. The output will be[1, 2, 3, 1, 2, 3, 1, 2, 3]
.
Extending the Repeating to Two Dimensions
Understand that
tile()
can also handle repetitions in multiple dimensions.Define the repetitions as a tuple: the first number for column-wise and the second for row-wise repetitions.
Apply
tile()
with the dimension tuple.python# Repeat the array in both row and column dimensions two_dimension_repetition = np.tile(a, (2, 3)) print(two_dimension_repetition)
Here, the array is repeated 3 times horizontally and 2 times vertically, resulting in a 2x9 matrix.
Advanced Applications of tile()
Using tile() with Two-dimensional Arrays
Take the example of a two-dimensional array.
Decide the repetition pattern for both dimensions.
Apply the
tile()
function accordingly.python# Create a two-dimensional array b = np.array([[1, 2], [3, 4]]) # Repeat the array repeated_two_dimension = np.tile(b, (2, 3)) print(repeated_two_dimension)
In this case,
tile()
repeats the2x2
matrix three times horizontally and two times vertically, resulting in a4x6
matrix.
Handling Higher Dimensions
Realize that
tile()
is not limited to repeating arrays just in 1 or 2 dimensions.Specify the repetition pattern for higher dimensions as needed.
Execute the
tile()
function to see the effect in higher dimensions.pythonc = np.array([[[1, 2], [3, 4]]]) # Specifying repetitions for 3D repeated_three_dimension = np.tile(c, (2, 2, 2)) print(repeated_three_dimension)
This will result in each element of the 3-dimensional array
c
being repeated according to the specified pattern, effectively demonstrating howtile()
accommodates more complex, multi-dimensional repetition schemes.
Conclusion
Utilizing the tile()
function in Numpy enhances your ability to manipulate and expand arrays in Python efficiently. Whether dealing with simple one-dimensional arrays or complex multi-dimensional ones, tile()
offers an effective way to repeat elements across any specified dimensions. This technique simplifies the process of data replication and can be particularly powerful in numerical simulations, data augmentation for machine learning, or any scenario requiring repeated array patterns. With the concepts and examples provided, adeptly employ tile()
to make your array handling tasks more robust and streamlined.
No comments yet.