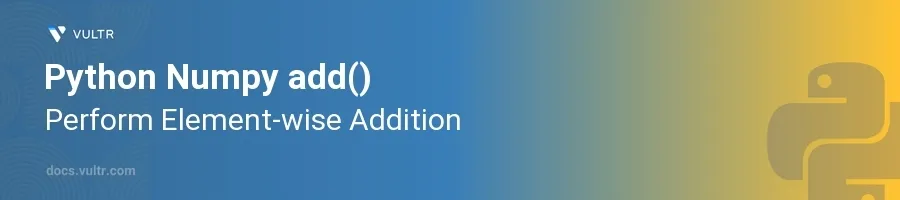
Introduction
The numpy.add()
function in Python is essential for performing element-wise addition across arrays, which is a common task in numerical and scientific computing. This function is part of the NumPy library, a fundamental package for numerical computations in Python. It offers a reliable way to execute addition operations on array-like structures efficiently and effectively.
In this article, you will learn how to leverage the numpy.add()
function to perform element-wise addition on various array types and structures. Discover different application scenarios ranging from simple numeric additions to handling more complex multidimensional arrays.
Simplifying Addition of Two Arrays
Adding Two One-Dimensional Arrays
Import the NumPy library.
Initialize two one-dimensional arrays of equal length.
Use
numpy.add()
to perform the addition.pythonimport numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) result = np.add(array1, array2) print(result)
This script outputs the element-wise addition of
array1
andarray2
, resulting in[5 7 9]
.
Adding Arrays with Broadcasting
Understand that NumPy supports broadcasting smaller arrays to match the dimensions of a larger one.
Define a scalar and a one-dimensional array.
Apply
numpy.add()
to observe broadcasting in action.pythonscalar = 5 array = np.array([1, 2, 3]) result = np.add(scalar, array) print(result)
The scalar value is broadcasted and added to each element of
array
, producing[6 7 8]
.
Working with Multidimensional Arrays
Add Two Dimensional Arrays
Create two two-dimensional arrays.
Use
numpy.add()
for adding them element-wise.pythonarray1 = np.array([[1, 2], [3, 4]]) array2 = np.array([[5, 6], [7, 8]]) result = np.add(array1, array2) print(result)
Here,
numpy.add()
adds each corresponding element of the two matrices, resulting in[[6 8] [10 12]]
.
Handling Arrays of Different Shapes
Learn about handling non-aligned arrays using broadcasting rules.
Combine a two-dimensional array and one-dimensional array using
numpy.add()
.pythonmat = np.array([[1, 2], [3, 4]]) vec = np.array([1, 1]) result = np.add(mat, vec) print(result)
The vector
vec
is broadcasted across each row of the matrixmat
, thereby adding1
to each element, which results in[[2 3] [4 5]]
.
Conclusion
The numpy.add()
function is a powerful tool for efficient and flexible element-wise addition of arrays in Python. Handle both simple and complex data structures proficiently by employing broadcasting when necessary. Use this function to simplify data manipulation in scientific computing, data analysis, and machine learning projects. Implement the techniques discussed to optimize and fine-tune array operations, ensuring your programs are both effective and efficient.
No comments yet.